如何快速編寫增刪改程序?C/C 鏈表操作,基礎數據結構的使用
源碼:
#define _CRT_SECURE_NO_WARNINGS
#include
#include
#include
struct student
{
char name[20];
int age;
int num;
};
//單鏈表的結構體
typedef struct SingleList
{
struct student mystudent;
//指針域
struct SingleList *next;
}LIST, *LPLIST;
/*
別名:習慣大寫
起別名---小名
//好處:單詞少,好看(含義更精簡)
struct SingleList 換一種叫法: LIST;
strcut SingleList * 換一種叫法: LPLIST
*/
//------->2.創建鏈表 ---任何結構都需要用一個東西去表示
LPLIST CreateList()
{
//創建過程就是初始化過程---初始化基本數據成員過程
//需要內存空間
LPLIST List = (LPLIST)malloc(sizeof(LIST));
if (List == nullptr)
{
printf("失敗了");
system("pause");
exit(0);
}
//初始化基本數據成員----有表頭的鏈表
List->next = nullptr;
return List;
}
//------->3.創建結點
LPLIST CreateNode(struct student mystudent)
{
//1.需要內存
LPLIST Node = (LPLIST)malloc(sizeof(LIST));
//2.初始化基本數據成員
strcpy(Node->mystudent.name, mystudent.name);
Node->mystudent.age = mystudent.age;
Node->mystudent.num = mystudent.num;
Node->next = nullptr;
return Node;
}
//------->4.2 尾插法
void InsertListTailNode(LPLIST List, struct student mystudent)
{
//找到表尾--->定義一個移動的指針
LPLIST tailNode = List;
while (tailNode->next != nullptr)
{
tailNode = tailNode->next;
}
//創建插入的結點
LPLIST newNode = CreateNode(mystudent);
tailNode->next = newNode;
}
//------->5.判斷是否為空
//和創建的時候比較
int IsEmptyList(LPLIST List)
{
if (List->next == nullptr)
return 1; //返回1表示為空
return 0; //表示不為空
}
////------->6.列印數據
void PrintList(LPLIST List)
{
if (IsEmptyList(List))
{
printf("鏈表為空,無法列印");
system("pause");
exit(0);
}
LPLIST pNext = List->next;
while (pNext != nullptr)
{
printf("姓名:%s年齡:%d編號:%d", pNext->mystudent.name, pNext->mystudent.age, pNext->mystudent.num);
pNext = pNext->next;
}
}
//------->9.按照編號指定位置刪除
void DeleteListAppoinNode_num(LPLIST List, int num)
{
//創建兩個移動的指針:去找指定位置和指定位置的前面
LPLIST frontNode = List;
//frontNode->next==taiNode:判斷相鄰
LPLIST tailNode = List->next;
//判斷是否為空
while (tailNode->mystudent.num != num)
{
/*
frontNode=frontNode->next;
tailNode=tailNode->next;
*/
frontNode = tailNode;
tailNode = frontNode->next;
if (tailNode == nullptr)
{
printf("未找到指定位置");
system("pause");
exit(0);
}
}
frontNode->next = tailNode->next;
free(tailNode);
}
//------->9.按照姓名指定位置刪除
void DeleteListAppoinNode_name(LPLIST List, char *name)
{
//創建兩個移動的指針:去找指定位置和指定位置的前面
LPLIST frontNode = List;
//frontNode->next==taiNode:判斷相鄰
LPLIST tailNode = List->next;
//判斷是否為空
while (strcmp(tailNode->mystudent.name, name))
{
/*
frontNode=frontNode->next;
tailNode=tailNode->next;
*/
frontNode = tailNode;
tailNode = frontNode->next;
if (tailNode == nullptr)
{
printf("未找到指定位置");
system("pause");
exit(0);
}
}
frontNode->next = tailNode->next;
free(tailNode);
}
void Menu()
{
printf("1.錄入信息");
printf("2.刪除信息");
printf("3.瀏覽信息");
}
LPLIST List = CreateList(); //List創建成功
void key_down()
{
char name[20] = "";
int num = 0;
fflush(stdin);
char choice = getchar();
int DChoice = 1;
switch (choice)
{
case 1 :
system("cls");
struct student mystudent;
printf("請輸入:姓名:年齡:編號:");
scanf("%s%d%d", mystudent.name, &mystudent.age, &mystudent.num);
InsertListTailNode(List, mystudent);
break;
case 2 :
system("cls");
fflush(stdin);
printf("1.按照姓名刪除");
printf("2.按照編號");
DChoice = getchar();
switch (DChoice)
{
case 1 :
printf("請輸入要刪除的姓名:");
scanf("%s", name);
DeleteListAppoinNode_name(List, name);
break;
case 2 :
printf("請輸入要刪除的編號:");
scanf("%s", name);
DeleteListAppoinNode_num(List, num);
}
break;
case 3 :
system("cls");
printf("學生信息:");
PrintList(List);
break;
default:
printf("輸入錯誤");
system("pause");
}
}

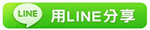
※學了這麼久,這些選擇題你會嗎?
※C 標準輸入輸出流的控制符
※《實用C》第4課:玩轉 Hello World!
※程序員的突發奇想,老闆竟把他炒了,原因是思想太超前
TAG:maye |