Tkinter小部件用法介紹
前些時候,小編給大家簡單地分享了Tkinter內置模塊中小部件的創建方式。今天,我將深入地給大家分享Tkinter中各個小部件的用法。
一、Tkinter部件參數說明
上次,《設計一個界面,很簡單》文章裡面說明了:Tkinter模塊只是Tcl/Tk的包裝器。因此,我們創建的小部件參數都可以從Tcl/Tk文檔中查閱,其鏈接如下:http://www.tcl.tk/man/tcl8.6/TkCmd/contents.htm。當然,你也可以從Python官方文檔:《Graphical User Interfaces with Tk》查閱各個小部件的參數信息,其鏈接如下:https://docs.python.org/3.5/library/tk.html。
二、創建Tkinter部件
首先,我們使用以下三行代碼創建一個頂級窗體,如下所示:
import tkinter as tk
root = tk.Tk()
root.mainloop()
如果你想要在頂級窗體上添加一個標題,只需要添加一行代碼,如下所示:
root.title("Tkinter Widget")
以上代碼顯示界面如下:
下面創建小部件的內容將省略以上四行代碼。
2.1 標籤Label
我們將創建一個名為"This is a Label"的標籤,其代碼如下:
tk.Label(root, text="This is a Label").pack()
如果你希望創建出來的小部件更加美觀,請使用以下代碼:
from tkinter import ttk
ttk.Label(root, text="This is a Label").pack()
如果你想對Label做更加細微的設置,請參見下面的參數:
activebackground, activeforeground, anchor, background, bitmap, borderwidth, cursor, disabledforeground, font, foreground, highlightbackground, highlightcolor, highlightthickness, image, justify, padx, pady, relief, takefocus, text, textvariable, underline, wraplength, height, state, width。
各參數含義請參見Tcl/Tk官方文檔。另外,下面的代碼將優先使用ttk裡面的小部件。
2.2 按鈕Button
我們創建一個名為"This is a Button"的按鈕,當點擊該按鈕時,改變標籤的文本顏色及內容,同時修改Button按鈕的文字內容。代碼如下:
def Change():
label.config(text="This is a Red Label", foreground="red")
button.config(text="Clicked Button")
label = ttk.Label(root, text="A Label")
button = ttk.Button(root, text="This is A Button", command=Change)
label.pack()
button.pack()
點擊按鈕前的界面:
點擊按鈕後的界面:
如果你想對Button做更加細微的設置,請參見下面的參數:
activebackground, activeforeground, anchor, background, bitmap, borderwidth, cursor, disabledforeground, font, foreground highlightbackground, highlightcolor, highlightthickness, image, justify, padx, pady, relief, repeatdelay, repeatinterval, takefocus, text, textvariable, underline, wraplength。
各參數含義請參見Tcl/Tk官方文檔。
2.3 文本框Entry
我們創建一個文本框,然後輸入任意文本,當點擊按鈕時,輸入的文本信息變成按鈕的文本信息。如下所示:
def Change():
button.config(text=text.get())
text = tk.StringVar()
ttk.Label(root, text="Enter several text:").grid(column=0, row=0)
textbox = ttk.Entry(root, width=15, textvariable=text)
button = ttk.Button(root, text="Click", command=Change)
textbox.grid(column=0, row=1)
button.grid(column=1, row=1)
點擊按鈕前的界面:
點擊按鈕後的界面:
如果你想對Entry做更加細微的設置,請參見下面的參數:
background, bd, bg, borderwidth, cursor, exportselection, fg, font, foreground, highlightbackground, highlightcolor, highlightthickness, insertbackground,
insertborderwidth, insertofftime, insertontime, insertwidth, invalidcommand, invcmd, justify, relief, selectbackground, selectborderwidth, selectforeground, show, state, takefocus, textvariable, validate, validatecommand, vcmd, width,
xscrollcommand.
各參數含義請參見Tcl/Tk官方文檔。
2.4 組合框Combobox
我們分別創建一個文本框和組合框,輸入任意文本,然後輸入數字或者選擇下拉選項的數字,選擇界面時,游標聚焦在文本框,當單擊按鈕後,輸入的信息成為按鈕的文本信息,同時,禁用按鈕的顯示狀態。
def Change():
button.config(text=text.get() + number.get() , state="disabled")
text = tk.StringVar()
number = tk.StringVar()
ttk.Label(root, text="Enter several text:").grid(column=0, row=0)
ttk.Label(root, text="Choose a number:").grid(column=1, row=0)
textbox = ttk.Entry(root, width=15, textvariable=text)
button = ttk.Button(root, text="Click", command=Change)
combobox = ttk.Combobox(root, width=15, textvariable=number)
textbox.focus()
textbox.grid(column=0, row=1)
combobox["values"] = (1, 2, 4, 8, 16, 32)
combobox.current(0)
combobox.grid(column=1, row=1)
button.grid(column=2, row=1)
root.mainloop()
運行程序並選擇界面:
輸入文本並選擇數字後的界面:
如果你想對Combobox做更加細微的設置,請參見下面的參數:
class, cursor, style, takefocus, exportselection, justify, height, postcommand, state, textvariable, values, width。
各參數含義請參見Tcl/Tk官方文檔。
2.5 複選框Checkbutton
接著上面組合框的示例,我們將創建三種不同狀態的複選框:
在上面代碼的基礎上,增加以下代碼創建出下圖的複選框:
chk_dis = tk.IntVar()
chk_des = tk.IntVar()
chk_sel = tk.IntVar()
chk1 = tk.Checkbutton(root, text="Disabled", variable=chk_dis, state="disabled")
chk2 = tk.Checkbutton(root, text="Deselect", variable=chk_des)
chk3 = tk.Checkbutton(root, text="Select", variable=chk_sel)
chk1.select()
chk2.deselect()
chk3.select()
chk1.grid(column=0, row=4, sticky=tk.W)
chk2.grid(column=1, row=4, sticky=tk.W)
chk3.grid(column=2, row=4, sticky=tk.W)
如果你想對Checkbutton做更加細微的設置,請參見下面的參數:
activebackground, activeforeground, anchor, background, bd, bg, bitmap, borderwidth, command, cursor, disabledforeground, fg, font, foreground, height, highlightbackground, highlightcolor, highlightthickness, image, indicatoron, justify, offvalue, onvalue, padx,
pady, relief, selectcolor, selectimage, state, takefocus, text, textvariable, underline, varable, width, wraplength.
各參數含義請參見Tcl/Tk官方文檔。
2.6 單選按鈕Radiobutton
接著上面複選框的示例,我們將創建三個單選按鈕,當選擇任意一個單選按鈕時,將主窗體背景空隙填充不同的顏色。
在上面代碼的基礎上,增加以下代碼創建出下圖的單選按鈕:
def Colorselector():
color = rad.get()
if color == 1:
root.config(background="RED")
elif color == 2:
root.config(background="GREEN")
elif color ==3:
root.config(background="BLUE")
rad = tk.IntVar()
rad1 = tk.Radiobutton(root, text="RED", variable=rad, value=1, command=Colorselector)
rad2 = tk.Radiobutton(root, text="GREEN", variable=rad, value=2, command=Colorselector)
rad3 = tk.Radiobutton(root, text="BLUE", variable=rad, value=3, command=Colorselector)
rad1.grid(column=0, row=5, sticky=tk.W)
rad2.grid(column=1, row=5, sticky=tk.W)
rad3.grid(column=2, row=5, sticky=tk.W)
運行時的界面:
選擇第一個單選按鈕的界面:
如果你想對Radiobutton做更加細微的設置,請參見下面的參數:
activebackground, activeforeground, anchor, background, bd, bg, bitmap, borderwidth, command, cursor, disabledforeground, fg, font, foreground, height, highlightbackground, highlightcolor, highlightthickness, image, indicatoron, justify, padx, pady, relief, selectcolor, selectimage, state, takefocus, text, textvariable, underline, value, variable, width, wraplength.
各參數含義請參見Tcl/Tk官方文檔。
以上內容簡單地介紹了Tkinter模塊中主要小部件的用法,希望對大家有所幫助。

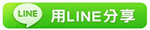
TAG:Python和Office之家 |