JD Stock-股價數據分析01
嗯!貌似簡易教程更受大家的歡迎哦~今天就繼續分享一下Python的簡易實踐內容,因為也是工作日,只能晚上抽空簡單寫一下,內容也不多,大家也好消化消化。(我還是用Python3)
本次實踐的對象是股價,通過Python對股價進行簡易的數據分析,從中可以對pandas庫、numpy庫、matplotlib庫進行回顧學習哦!
但是,正所謂「授人以魚不如授人以漁」,我還是簡單說一下如何自己下載數據集吧。
如何從雅虎finance下載股價數據?
Step1:登錄雅虎finance:https://finance.yahoo.com/
Step2:在檢索欄搜索你想要下載的公司(如JD)
Step3:點擊historical data(歷史數據)
Step4:選擇time period(時間跨度),點擊download即可完成數據集的下載。
好了,進入正題,進行簡易的股價數據分析。
1)首先是讀入數據。
import pandas as pd
import numpy as np
#讀取數據,必須這樣做,才可以將日期作為索引,而且是datetime的索引
stock= pd.read_csv("/JD.csv",index_col=[0],parse_dates = True)
stock_1=stock[1:30]
stock.head()
讓我們簡單說一下數據內容吧。
Open是當天的開始價格(不是前一天閉市的價格);
High是股票當天的最高價;
Low是股票當天的最低價;
Close是閉市時間的股票價格。
Adjust close是根據法人行為調整之後的閉市價格;
Volume指交易數量。
2)可視化股價數據
import matplotlib.pyplot as plt # Import matplotlib
# This line is necessary for the plot to appear in a Jupyter notebook
%matplotlib inline
# Control the default size of figures in this Jupyter notebook
%pylab inline
pylab.rcParams["figure.figsize"] = (15, 9) # Change the size of plots
stock["Close"].plot(grid = True)# Plot the adjusted closing price of JD
3)畫K線圖
玩過股票的人都知道K線圖。相傳K線圖起源於日本德川幕府時代,當時的商人用此圖來記錄米市的行情和價格波動,後來K線圖被引入到股票市場。每天的四項指標數據用如下蠟燭形狀的圖形來記錄,不同的顏色代表漲跌情況。
(網路圖片,侵刪)
下面用Python的Matplotlib.finance模塊來實現K線圖的繪製。下面我要粘貼一大段代碼,代碼的內容都是在設置坐標軸的格式的(自己可以細細研究哦~)
from matplotlib.dates import DateFormatter, WeekdayLocator,
DayLocator, MONDAY
from matplotlib.finance import candlestick_ohlc
def pandas_candlestick_ohlc(dat, stick = "day", otherseries = None):
"""
:param dat: pandas DataFrame object with datetime64 index, and float columns "Open", "High", "Low", and "Close", likely created via DataReader from "yahoo"
:param stick: A string or number indicating the period of time covered by a single candlestick. Valid string inputs include "day", "week", "month", and "year", ("day" default), and any numeric input indicates the number of trading days included in a period
:param otherseries: An iterable that will be coerced into a list, containing the columns of dat that hold other series to be plotted as lines
This will show a Japanese candlestick plot for stock data stored in dat, also plotting other series if passed.
"""
mondays = WeekdayLocator(MONDAY) # major ticks on the mondays
alldays = DayLocator() # minor ticks on the days
dayFormatter = DateFormatter("%d") # e.g., 12
# Create a new DataFrame which includes OHLC data for each period specified by stick input
transdat = dat.loc[:,["Open", "High", "Low", "Close"]]
if (type(stick) == str):
if stick == "day":
plotdat = transdat
stick = 1 # Used for plotting
elif stick in ["week", "month", "year"]:
if stick == "week":
transdat["week"] = pd.to_datetime(transdat.index).map(lambda x: x.isocalendar()[1]) # Identify weeks
elif stick == "month":
transdat["month"] = pd.to_datetime(transdat.index).map(lambda x: x.month) # Identify months
transdat["year"] = pd.to_datetime(transdat.index).map(lambda x: x.isocalendar()[0]) # Identify years
grouped = transdat.groupby(list(set(["year",stick]))) # Group by year and other appropriate variable
plotdat = pd.DataFrame({"Open": [], "High": [], "Low": [], "Close": []}) # Create empty data frame containing what will be plotted
for name, group in grouped:
plotdat = plotdat.append(pd.DataFrame({"Open": group.iloc[0,0],
"High": max(group.High),
"Low": min(group.Low),
"Close": group.iloc[-1,3]},
index = [group.index[0]]))
if stick == "week": stick = 5
elif stick == "month": stick = 30
elif stick == "year": stick = 365
elif (type(stick) == int and stick >= 1):
transdat["stick"] = [np.floor(i / stick) for i in range(len(transdat.index))]
grouped = transdat.groupby("stick")
plotdat = pd.DataFrame({"Open": [], "High": [], "Low": [], "Close": []}) # Create empty data frame containing what will be plotted
for name, group in grouped:
plotdat = plotdat.append(pd.DataFrame({"Open": group.iloc[0,0],
"High": max(group.High),
"Low": min(group.Low),
"Close": group.iloc[-1,3]},
index = [group.index[0]]))
else:
raise ValueError("Valid inputs to argument "stick" include the strings "day", "week", "month", "year", or a positive integer")
# Set plot parameters, including the axis object ax used for plotting
fig, ax = plt.subplots()
fig.subplots_adjust(bottom=0.2)
if plotdat.index[-1] - plotdat.index[0] < pd.Timedelta("730 days"):
weekFormatter = DateFormatter("%b %d") # e.g., Jan 12
else:
weekFormatter = DateFormatter("%b %d, %Y")
ax.grid(True)
# Create the candelstick chart
plotdat["Low"].tolist(), plotdat["Close"].tolist())),
colorup = "black", colordown = "red", width = stick * .4)
# Plot other series (such as moving averages) as lines
if otherseries != None:
if type(otherseries) != list:
otherseries = [otherseries]
dat.loc[:,otherseries].plot(ax = ax, lw = 1.3, grid = True)
ax.xaxis_date()
ax.autoscale_view()
plt.setp(plt.gca().get_xticklabels(), rotation=45, horizontalalignment="right")
plt.show()
pandas_candlestick_ohlc(stock)
可以看到K線圖就畫好了,但是由於數據時間跨度太大,所以看不清,我這裡就選取了30天的數據,又畫了一個圖。
這個就清楚多了,這裡紅色代表上漲,綠色代表下跌。
參考資料:

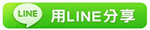
TAG:SAMshare |