python大師教你如何學習Requests
在給大家分享這個操作之前,小編引薦大家加下這個裙;四九一 三零八六五九,編號是;YZ,(務必填哦)大家遇到啥問題都可以在裡面交流,而且分享十年開發經歷牛人分享課一套,是個十分好的學習交流中央,也有程序員大神給大家熱心解答各種成績,很快滿員了,欲進從速哦,等大家參加學習交流基地呢
什麼是Requests
Requests是用python語言基於urllib編寫的,採用的是Apache2 Licensed開源協議的HTTP庫
如果你看過上篇文章關於urllib庫的使用,你會發現,其實urllib還是非常不方便的,而Requests它會比urllib更加方便,可以節約我們大量的工作。(用了requests之後,你基本都不願意用urllib了)一句話,requests是python實現的最簡單易用的HTTP庫,建議爬蟲使用requests庫。
默認安裝好python之後,是沒有安裝requests模塊的,需要單獨通過pip安裝
requests功能詳解
總體功能的一個演示
import requestsresponse = requests.get("https://www.baidu.com")print(type(response))print(response.status_code)print(type(response.text))print(response.text)print(response.cookies)print(response.content)print(response.content.decode("utf-8"))
我們可以看出response使用起來確實非常方便,這裡有個問題需要注意一下:
很多情況下的網站如果直接response.text會出現亂碼的問題,所以這個使用response.content
這樣返回的數據格式其實是二進位格式,然後通過decode()轉換為utf-8,這樣就解決了通過response.text直接返回顯示亂碼的問題.
請求發出後,Requests 會基於 HTTP 頭部對響應的編碼作出有根據的推測。當你訪問 response.text 之時,Requests 會使用其推測的文本編碼。你可以找出 Requests 使用了什麼編碼,並且能夠使用 response.encoding 屬性來改變它.如:
各種請求方式
requests里提供個各種請求方式
import requestsrequests.post("http://httpbin.org/post")requests.put("http://httpbin.org/put")requests.delete("http://httpbin.org/delete")requests.head("http://httpbin.org/get")requests.options("http://httpbin.org/get")
請求
基本GET請求
import requestsresponse = requests.get("http://httpbin.org/get")print(response.text)
帶參數的GET請求,例子1
import requestsresponse = requests.get("http://httpbin.org/get?name=zhaofan&age=23")print(response.text)
如果我們想要在URL查詢字元串傳遞數據,通常我們會通過httpbin.org/get?key=val方式傳遞。Requests模塊允許使用params關鍵字傳遞參數,以一個字典來傳遞這些參數,例子如下:
import requestsdata = { "name":"zhaofan", "age":22}response = requests.get("http://httpbin.org/get",params=data)print(response.url)print(response.text)
上述兩種的結果是相同的,通過params參數傳遞一個字典內容,從而直接構造url
注意:第二種方式通過字典的方式的時候,如果字典中的參數為None則不會添加到url上
解析json
import requestsimport jsonresponse = requests.get("http://httpbin.org/get")print(type(response.text))print(response.json())print(json.loads(response.text))print(type(response.json()))
從結果可以看出requests裡面集成的json其實就是執行了json.loads()方法,兩者的結果是一樣的
獲取二進位數據
在上面提到了response.content,這樣獲取的數據是二進位數據,同樣的這個方法也可以用於下載圖片以及
視頻資源
添加headers
和前面我們將urllib模塊的時候一樣,我們同樣可以定製headers的信息,如當我們直接通過requests請求知乎網站的時候,默認是無法訪問的
import requestsresponse =requests.get("https://www.zhihu.com")print(response.text)
這樣會得到如下的錯誤
因為訪問知乎需要頭部信息,這個時候我們在谷歌瀏覽器里輸入chrome://version,就可以看到用戶代理,將用戶代理添加到頭部信息
import requestsheaders = { "User-Agent":"Mozilla/5.0 (Macintosh; Intel Mac OS X 10_12_4) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36"}response =requests.get("https://www.zhihu.com",headers=headers)print(response.text)
這樣就可以正常的訪問知乎了
基本POST請求
通過在發送post請求時添加一個data參數,這個data參數可以通過字典構造成,這樣
對於發送post請求就非常方便
import requestsdata = { "name":"zhaofan", "age":23}response = requests.post("http://httpbin.org/post",data=data)print(response.text)
同樣的在發送post請求的時候也可以和發送get請求一樣通過headers參數傳遞一個字典類型的數據
響應
我們可以通過response獲得很多屬性,例子如下
結果如下:
狀態碼判斷
Requests還附帶了一個內置的狀態碼查詢對象
主要有如下內容:
100: ("continue",),
101: ("switching_protocols",),
102: ("processing",),
103: ("checkpoint",),
122: ("uri_too_long", "request_uri_too_long"),
200: ("ok", "okay", "all_ok", "all_okay", "all_good", "o/", ""),
201: ("created",),
202: ("accepted",),
203: ("non_authoritative_info", "non_authoritative_information"),
204: ("no_content",),
205: ("reset_content", "reset"),
206: ("partial_content", "partial"),
207: ("multi_status", "multiple_status", "multi_stati", "multiple_stati"),
208: ("already_reported",),
226: ("im_used",),
Redirection.
300: ("multiple_choices",),
301: ("moved_permanently", "moved", "o-"),
302: ("found",),
303: ("see_other", "other"),
304: ("not_modified",),
305: ("use_proxy",),
306: ("switch_proxy",),
307: ("temporary_redirect", "temporary_moved", "temporary"),
308: ("permanent_redirect",
"resume_incomplete", "resume",), # These 2 to be removed in 3.0
Client Error.
400: ("bad_request", "bad"),
401: ("unauthorized",),
402: ("payment_required", "payment"),
403: ("forbidden",),
404: ("not_found", "-o-"),
405: ("method_not_allowed", "not_allowed"),
406: ("not_acceptable",),
407: ("proxy_authentication_required", "proxy_auth", "proxy_authentication"),
408: ("request_timeout", "timeout"),
409: ("conflict",),
410: ("gone",),
411: ("length_required",),
412: ("precondition_failed", "precondition"),
413: ("request_entity_too_large",),
414: ("request_uri_too_large",),
415: ("unsupported_media_type", "unsupported_media", "media_type"),
416: ("requested_range_not_satisfiable", "requested_range", "range_not_satisfiable"),
417: ("expectation_failed",),
418: ("im_a_teapot", "teapot", "i_am_a_teapot"),
421: ("misdirected_request",),
422: ("unprocessable_entity", "unprocessable"),
423: ("locked",),
424: ("failed_dependency", "dependency"),
425: ("unordered_collection", "unordered"),
426: ("upgrade_required", "upgrade"),
428: ("precondition_required", "precondition"),
429: ("too_many_requests", "too_many"),
431: ("header_fields_too_large", "fields_too_large"),
444: ("no_response", "none"),
449: ("retry_with", "retry"),
450: ("blocked_by_windows_parental_controls", "parental_controls"),
451: ("unavailable_for_legal_reasons", "legal_reasons"),
499: ("client_closed_request",),
Server Error.
500: ("internal_server_error", "server_error", "/o", ""),
501: ("not_implemented",),
502: ("bad_gateway",),
503: ("service_unavailable", "unavailable"),
504: ("gateway_timeout",),
505: ("http_version_not_supported", "http_version"),
506: ("variant_also_negotiates",),
507: ("insufficient_storage",),
509: ("bandwidth_limit_exceeded", "bandwidth"),
510: ("not_extended",),
511: ("network_authentication_required", "network_auth", "network_authentication"),
通過下面例子測試:(不過通常還是通過狀態碼判斷更方便)

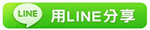
TAG:燕子教你學Python |