mybatis 多數據配置和切換
這裡以在項目里配置dataSource、dataSource_order、dataSource_mycat這三個數據源為例。
1.首先要在mybatis的配置文件里配置這三個數據源的地址、用戶名、密碼等相關信息,這一步和單個數據源是一致的。
實例代碼如下(我的mybatis配置文件為spring-mybatis.xml):
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource"
init-method="init" destroy-method="close">
<property name="url" value="jdbc:mysql://127.0.0.1:3306/test_office?useUnicode=true&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="test"/>
</bean>
<bean id="dataSource_order" class="com.alibaba.druid.pool.DruidDataSource"
init-method="init" destroy-method="close">
<property name="url" value="jdbc:mysql://192.168.1.1:3306/test?useUnicode=true&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="test"/>
</bean>
<bean id="dataSource_mycat" class="com.alibaba.druid.pool.DruidDataSource"
init-method="init" destroy-method="close">
<property name="url" value="jdbc:mysql://192.168.1.2:3306/TESTDB?useUnicode=true&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</bean>
2.在項目中加入以下幾個類文件
具體代碼如下:DataSourceAdvice
import com.beefly.office.constant.Constant;
public class DataSourceAdvice {
public void sysDataSource() {
DataSourceContextHolder.setDbType(Constant.SYS_DATA_SOURCE);
System.err.println(DataSourceContextHolder.getDbType());
}
public void orderDataSource() {
DataSourceContextHolder.setDbType(Constant.ORDER_DATA_SOURCE);
System.err.println(DataSourceContextHolder.getDbType());
}
public void mycatDataSource() {
DataSourceContextHolder.setDbType(Constant.MYCAT_DATA_SOURCE);
System.err.println(DataSourceContextHolder.getDbType());
}
}
DataSourceContextHolder代碼如下:
public class DataSourceContextHolder {
private static final ThreadLocal<String> contextHolder = new ThreadLocal<String>();
public static void setDbType(String dbType) {
contextHolder.set(dbType);
}
public static String getDbType() {
return ((String) contextHolder.get());
}
public static void clearDbType() {
contextHolder.remove();
}
}
DynamicDataSource代碼如下:
import java.util.logging.Logger;
import org.springframework.jdbc.datasource.lookup.AbstractRoutingDataSource;
public class DynamicDataSource extends AbstractRoutingDataSource {
@Override
public Logger getParentLogger() {
return null;
}
@Override
protected Object determineCurrentLookupKey() {
return DataSourceContextHolder.getDbType();
}
}
SqlSessionFactoryBeanHandler代碼如下:
import java.io.IOException;
import org.apache.ibatis.executor.ErrorContext;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.springframework.core.NestedIOException;
public class SqlSessionFactoryBeanHandler extends SqlSessionFactoryBean {
@Override
protected SqlSessionFactory buildSqlSessionFactory() throws IOException {
// TODO Auto-generated method stub
try {
return super.buildSqlSessionFactory();
} catch (NestedIOException e) {
e.printStackTrace(); // XML 有錯誤時列印異常。
throw new NestedIOException("Failed to parse mapping resource: "" + """, e);
} finally {
ErrorContext.instance().reset();
}
}
}
3.在mybatis配置文件中加入動態數據源切換的bean
<bean id ="dynamicSource" class= "com.beefly.office.handler.DynamicDataSource">
<property name ="targetDataSources">
<map key-type ="java.lang.String">
<entry value-ref ="dataSource" key= "dataSource"></entry>
<entry value-ref ="dataSource_order" key= "dataSource_order"></entry>
<entry value-ref ="dataSource_mycat" key= "dataSource_mycat"></entry>
</map>
</property>
<property name ="defaultTargetDataSource" ref= "dataSource"></property> <!-- 默認使用dataSource的數據源 -->
</bean >
4.加入一個aop實現切換
<aop:config>
<aop:aspect ref="dataSourceRoute">
<aop:pointcut expression ="execution(* com.beefly.office.dao.cxDao.*.*(..))" id= "pointcut" />
<aop:pointcut expression ="execution(* com.beefly.office.dao.mycatDao.*.*(..))" id= "pointcut_mycat" />
<aop:before method="orderDataSource" pointcut-ref="pointcut"/>
<aop:after method="sysDataSource" pointcut-ref="pointcut"/>
<aop:before method="mycatDataSource" pointcut-ref="pointcut_mycat"/>
<aop:after method="sysDataSource" pointcut-ref="pointcut_mycat"/>
</aop:aspect>
</aop:config>
5.全部mybatis配置為:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:cache="http://www.springframework.org/schema/cache"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.0.xsd">
<!-- <bean id="zkDataSource" class="com.beefly.common.jdbc.ZkDataSource" init-method="init" destroy-method="close">
<property name="connectProperties" value="_jdbc_config" />
</bean> -->
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource"
init-method="init" destroy-method="close">
<property name="url" value="jdbc:mysql://127.0.0.1:3306/test_office?useUnicode=true&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="test"/>
</bean>
<bean id="dataSource_order" class="com.alibaba.druid.pool.DruidDataSource"
init-method="init" destroy-method="close">
<property name="url" value="jdbc:mysql://192.168.1.1:3306/test?useUnicode=true&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="test"/>
</bean>
<bean id="dataSource_mycat" class="com.alibaba.druid.pool.DruidDataSource"
init-method="init" destroy-method="close">
<property name="url" value="jdbc:mysql://192.168.1.2:3306/TESTDB?useUnicode=true&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</bean>
<bean id ="dynamicSource" class= "com.beefly.office.handler.DynamicDataSource">
<property name ="targetDataSources">
<map key-type ="java.lang.String">
<entry value-ref ="dataSource" key= "dataSource"></entry>
<entry value-ref ="dataSource_order" key= "dataSource_order"></entry>
<entry value-ref ="dataSource_mycat" key= "dataSource_mycat"></entry>
</map>
</property>
<property name ="defaultTargetDataSource" ref= "dataSource"></property> <!-- 默認使用dataSource的數據源 -->
</bean >
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="configLocation" value="classpath:mybatis/mybatis-config.xml" />
<property name="mapperLocations" value="classpath:mapper/*.xml" />
<property name="dataSource" ref="dynamicSource" />
<property name="typeAliasesPackage">
<value>
com.beefly.office.domain
</value>
</property>
</bean>
<bean class ="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name ="basePackage" value= "com.beefly.office.dao"></property >
</bean>
<bean id="dataSourceRoute" class="com.beefly.office.handler.DataSourceAdvice"></bean>
<aop:config>
<aop:aspect ref="dataSourceRoute">
<aop:pointcut expression ="execution(* com.beefly.office.dao.cxDao.*.*(..))" id= "pointcut" />
<aop:pointcut expression ="execution(* com.beefly.office.dao.mycatDao.*.*(..))" id= "pointcut_mycat" />
<aop:before method="orderDataSource" pointcut-ref="pointcut"/>
<aop:after method="sysDataSource" pointcut-ref="pointcut"/>
<aop:before method="mycatDataSource" pointcut-ref="pointcut_mycat"/>
<aop:after method="sysDataSource" pointcut-ref="pointcut_mycat"/>
</aop:aspect>
</aop:config>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dynamicSource" />
</bean>
<!-- enable transaction demarcation with annotations -->
<tx:annotation-driven transaction-manager="transactionManager" />
<!-- enable transaction demarcation with annotations -->
<tx:annotation-driven />
</beans>

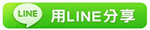
※json與protobuf的速度之爭
※DeepLearning-Ng編程中遇到的一些問題
TAG:程序員小新人學習 |