代碼實現順序表的操作函數
知識
06-23
[html] view plain copy
- #pragma once
- #include
<assert.h>
- #include
<stdio.h>
- typedef int DataType;
- #define MAX_SIZE (100)
- typedef struct SeqList{
- DataType array[MAX_SIZE];
- int size;
- }SeqList;
- //初始化
- void SeqListInit(SeqList *pSL);
- //銷毀
- void SeqListDestroy(SeqList *pSL);
- //增、刪、改、查
- //增
- //尾插
- void SeqListPushBack(SeqList *pSL, DataType data);
- //頭插
- void SeqListPushBack(SeqList *pSL, DataType data);
- //根據下標插入
- void SeqListInsert(SeqList *pSL, int pos, DataType data);
- //刪
- //尾刪
- void SeqListPopBack(SeqList *pSL);
- //頭刪
- void SeqListPopFront(SeqList *pSL);
- //根據下標刪除
- void SeqListErase(SeqList *pSL);
- //根據數據刪除,只刪除遇到的第一個
- void SeqListRemove(SeqList *pSL, DataType data);
- //查詢
- //返回遇到的第一個下標,如果沒有遇到,則返回-1
- int SeqListFind(SeqList *pSL, DataType data);
- //根據下標更新
- void SeqListUpdate(SeqList *pSL, int pos, DataType data);
- //初始化
- void SeqListInit(SeqList *pSL)
- {
- assert(pSL != NULL);
- pSL-
>
size = 0; - }
- //銷毀
- void SeqListDestroy(SeqList *pSL)
- {
- assert(pSL != NULL);
- pSL-
>
size = 0;
- }
- //尾插
- void SeqListPushBack(SeqList *pSL, DataType data)
- {
- assert(pSL != NULL);
- assert(pSL-
>
size<
MAX_SIZE
); - pSL-
>
array[pSL->
size] = data; - pSL-
>
size++; - }
- //頭插
- void SeqListPushFront(SeqList *pSL, DataType data)
- {
- assert(pSL != NULL);
- assert(pSL-
>
size<
MAX_SIZE
); - //1.以要搬移的數作為循環的指示
- int pos;
- for (pos = pSL-
>
size - 1; pos
>
=0; pos--) - {
- pSL-
>
array[pos + 1] = pSL->
array[pos]; - }
- pSL-
>
array[0] = data; - pSL-
>
size++; - }
- //根據下標插入
- void SeqListInsert(SeqList *pSL, int pos, DataType data)
- {
- assert(pSL != NULL);
- assert(pSL-
>
size<
MAX_SIZE
); - assert(pos
>
= 0 && pos<
= pSL-
>
size); - int space;
- for (space = pSL-
>
size; space>
pos; space--) - {
- pSL-
>
array[space] = pSL->
array[space - 1]; - }
- pSL-
>
array[pos] = data; - pSL-
>
size++; - }
- //刪
- //尾刪
- void SeqListPopBack(SeqList *pSL)
- {
- pSL-
>
size--; - }
- //頭刪
- void SeqListPopFront(SeqList *pSL)
- {
- assert(pSL != NULL);
- int pos;
- for (pos = 0; pos
<
pSL->
size-1; pos++)
- {
- pSL-
>
array[pos] = pSL->
array[pos + 1]; - }
- pSL-
>
size--; - }
- //根據下標刪除
- void SeqListErase(SeqList *pSL, int pos)
- {
- assert(pSL != NULL);
- assert(pos
>
= 0 && pos<
pSL->
size); - int node;
- for (node = pos; node
<
pSL->
size - 1; node++) - {
- pSL-
>
array[node] = pSL->
array[node + 1];
- }
- pSL-
>
size--; - }
- //查詢
- int SeqListFind(SeqList *pSL, DataType data)
- {
- assert(pSL != NULL);
- int i;
- for (i = 0; i
<
pSL->
size - 1; i++) - {
- if (pSL-
>
array[i] == data) - {
- return i;
- }
- }
- return -1;
- }
- //根據數據刪除
- void SeqListRemove(SeqList *pSL, DataType data)
- {
- int pos = SeqListFind(pSL, data);
- if (pos != -1) {
- // 如果找到了
- SeqListErase(pSL, pos);
- }
- }
- //根據下標更新
- void SeqListUpdate(SeqList *pSL, int pos, DataType data)
- {
- assert(pSL != NULL);
- assert(pos
>
= 0 && pos<
pSL->
size - 1); - pSL-
>
array[pos] = data; - }
- //列印函數
- void SeqListPrint(SeqList *pSL)
- {
- int i;
- for (i = 0; i
<
pSL->
size; i++) { - printf("%d ", pSL-
>
array[i]); - }
- printf("
"); - }
- void TestSeqList()
- {
- SeqList sl;
- SeqListInit(&sl);
- SeqListPushBack(&sl, 1);
- SeqListPushBack(&sl, 2);
- SeqListPushBack(&sl, 3);
- SeqListPushBack(&sl, 4);
- //SeqListPushFront(&sl, 0);
- //SeqListPushFront(&sl, -1);
- //SeqListInsert(&sl,2,2);
- //SeqListPopBack(&sl);
- //SeqListPopFront(&sl);
- //SeqListPopFront(&sl);
- //SeqListErase(&sl, 4);
- //SeqListRemove(&sl, 2);
- SeqListUpdate(&sl,1,6);
- SeqListPrint(&sl);
- }
用typedef int DataType; 對int 進行重命名。
定義一個結構體,結構體中參數為,一個DataType型的數組,一個DataType型的size,
數組中存放數據,size表示數組的長度。

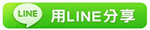
※Intel擬推動概率計算研究
※python腳本實現定時發送郵件
TAG:程序員小新人學習 |