Monkey可視化工具開發
在用monkey做android壓力測試時,一般都會選擇直接運行monkey命令的形式,由於重複性較強,我就寫了個monkey可視化運行的工具,monkey參數可自行調整。下面先附上效果圖:
以下為代碼:
1.cmd命令執行工具類
[java] view plain copy
import
java.io.BufferedReader;import
java.io.IOException;import
java.io.InputStreamReader;import
java.util.ArrayList;import
java.util.List;public
class
CmdUtils {- List<String>resultList=
new
ArrayList<String>(); public
List<String> excuteCmd(String command){- //String command="adb devices";
- String line =
null
;
- StringBuilder sb =
new
StringBuilder(); - Runtime runtime = Runtime.getRuntime();
try
{- Process process = runtime.exec(command);
- BufferedReader bufferedReader =
new
BufferedReader(new
InputStreamReader(process.getInputStream())); while
((line = bufferedReader.readLine()) !=null
) {- resultList.add(line);
- }
- }
catch
(IOException e) { - e.printStackTrace();
- }
return
resultList;- }
- }
2.獲取連接設備工具類
[java] view plain copy
import
java.io.BufferedReader;import
java.io.IOException;import
java.io.InputStreamReader;import
java.util.ArrayList;import
java.util.List;public
class
AndroidDevicesInfo {- List<String>devicesList=
new
ArrayList<String>(); public
List<String> getAndroidDevicesInfo(){- String command="adb devices";
- String line =
null
;
- StringBuilder sb =
new
StringBuilder(); - Runtime runtime = Runtime.getRuntime();
try
{- Process process = runtime.exec(command);
- BufferedReader bufferedReader =
new
BufferedReader(new
InputStreamReader(process.getInputStream())); while
((line = bufferedReader.readLine()) !=null
) {if
(line.contains("device")){if
(!line.contains("devices")){- String deviceId=line.substring(0, line.indexOf("device"));
- devicesList.add(deviceId);
- }
- }
- }
- }
catch
(IOException e) { - e.printStackTrace();
- }
return
devicesList;
- }
- }
3.載入指定設備的應用包集合
[java] view plain copy
package
MonkeyTool;import
java.io.BufferedReader;import
java.io.IOException;import
java.io.InputStreamReader;import
java.util.ArrayList;import
java.util.List;public
class
AndroidPackageInfo {
- List<String>packageList=
new
ArrayList<String>(); - //獲取指定設備下的包集合
public
List<String> getAndroidPackageInfo(String deviceId){- String command="adb -s "+deviceId+" shell pm list packages";
- String line =
null
; - StringBuilder sb =
new
StringBuilder(); - Runtime runtime = Runtime.getRuntime();
try
{- Process process = runtime.exec(command);
- BufferedReader bufferedReader =
new
BufferedReader(new
InputStreamReader(process.getInputStream())); while
((line = bufferedReader.readLine()) !=null
) {if
(line.contains("package")){
- //截取包字元串放入list中
- String packageItem=line.substring(line.indexOf("package")+8,line.length());
- //String packageItem=line;
- packageList.add(packageItem);
- }
- }
- }
catch
(IOException e) { - e.printStackTrace();
- }
return
packageList;- }
- }
4.載入設備線程
[java] view plain copy
package
MonkeyTool;import
java.util.List;import
javax.swing.JComboBox;- //載入設備任務
public
class
DevicesThreadextends
Thread{
- AndroidDevicesInfo androidDevicesInfo;
- Object obj;
- String[] s;
- JComboBox devicesComBox;
- List<String> list;
- String devicesId;
public
DevicesThread(JComboBox devicesComBox) {- // TODO Auto-generated constructor stub
this
.devicesComBox=devicesComBox;- //System.out.println("aa");
- }
- @Override
public
void
run() {- // TODO Auto-generated method stub
super
.run();- // TODO Auto-generated method stub
- devicesId=(String) devicesComBox.getSelectedItem();
- System.out.println(devicesId);
- androidDevicesInfo=
new
AndroidDevicesInfo(); - list=androidDevicesInfo.getAndroidDevicesInfo();
- devicesComBox.removeAllItems();
if
(list.size()==0){- devicesComBox.addItem("無設備連接");
- }
else
{for
(String item : list) {- System.out.println(item);
- devicesComBox.addItem(item);
- }
- }
- }
- }
[java] view plain copy
package
MonkeyTool;import
java.util.List;import
javax.swing.JComboBox;- //載入包名任務
public
class
PackageThreadextends
Thread {- AndroidDevicesInfo androidDevicesInfo;
- AndroidPackageInfo androidPackageInfo;
- Object obj;
- String[] s;
- JComboBox devicesComBox;
- JComboBox packageComBox;
- List<String> list;
- String devicesId;
public
PackageThread(JComboBox devicesComBox,JComboBox packageComBox,String devicesId) {- // TODO Auto-generated constructor stub
this
.devicesComBox=devicesComBox;this
.packageComBox=packageComBox;this
.devicesId=devicesId;- }
- @Override
public
void
run() {- // TODO Auto-generated method stub
super
.run();- // TODO Auto-generated method stub
- devicesId=(String) devicesComBox.getSelectedItem();
- System.out.println(devicesId);
- androidPackageInfo=
new
AndroidPackageInfo(); - list=androidPackageInfo.getAndroidPackageInfo(devicesId);
- packageComBox.removeAllItems();
if
(list.size()==0){- packageComBox.addItem("未識別應用包名");
- }
else
{for
(String item : list) {- System.out.println(item);
- packageComBox.addItem(item);
- }
- }
- }
- }
6.xml工具類
[java] view plain copy
package
MonkeyTool;import
java.io.File;import
java.io.FileReader;import
java.util.ArrayList;import
java.util.HashMap;import
java.util.List;import
java.util.Map;import
javax.xml.parsers.DocumentBuilder;import
javax.xml.parsers.DocumentBuilderFactory;import
javax.xml.parsers.ParserConfigurationException;import
org.w3c.dom.Document;import
org.w3c.dom.Element;import
org.w3c.dom.Node;import
org.w3c.dom.NodeList;- //xml解析類
public
class
ReadMonkeyConfig {- List<String>case_list;
- Map<String, String> map;
- List<HashMap<String, String>>monkeyArgs;
- //獲取id名
public
List XmlToList_id()throws
Exception {- case_list=
new
ArrayList<>(); - // File xmlFile = new File("src/Testcase.xml");
- // File xmlFile = new File("E:/YallaProject/Testcase.xml");
- File file=
new
File(""); - System.out.println(file.getAbsolutePath());
- File xmlFile=
new
File(file.getAbsolutePath()+"\MonkeyConfig.xml"); - DocumentBuilderFactory builderFactory = DocumentBuilderFactory.newInstance();
- DocumentBuilder builder = builderFactory.newDocumentBuilder();
- Document doc = builder.parse(xmlFile);
- doc.getDocumentElement().normalize();
- System.out.println("Root element: "+doc.getDocumentElement().getNodeName());
- NodeList nList = doc.getElementsByTagName("case");
for
(int
i = 0 ; i<nList.getLength();i++){- Node node = nList.item(i);
- System.out.println("Node name: "+ node.getNodeName());
- Element ele = (Element)node;
- // System.out.println("----------------------------");
if
(node.getNodeType() == Element.ELEMENT_NODE){- case_list.add(ele.getAttribute("id"));
- }
- }
return
case_list;- }
- //根據id名獲取各項配置
public
List getConfigData(String args)throws
Exception {- map=
new
HashMap<String,String>(); - List<Map<String, String>> list =
new
ArrayList<Map<String, String>>();; - File file=
new
File(""); - System.out.println(file.getAbsolutePath());
- File xmlFile=
new
File(file.getAbsolutePath()+"\MonkeyConfig.xml"); - DocumentBuilderFactory builderFactory = DocumentBuilderFactory.newInstance();
- DocumentBuilder builder = builderFactory.newDocumentBuilder();
- Document doc = builder.parse(xmlFile);
- doc.getDocumentElement().normalize();
- System.out.println("Root element: "+doc.getDocumentElement().getNodeName());
- NodeList nList = doc.getElementsByTagName("case");
for
(int
i = 0 ; i<nList.getLength();i++){- Node node = nList.item(i);
- System.out.println("Node name: "+ node.getNodeName());
- Element ele = (Element)node;
if
(node.getNodeType() == Element.ELEMENT_NODE){if
(ele.getAttribute("id").equals(args)){- map.put("touchEvent", ele.getElementsByTagName("touchEvent").item(0).getTextContent());
- map.put("motionEvent", ele.getElementsByTagName("motionEvent").item(0).getTextContent());
- map.put("switchEvent", ele.getElementsByTagName("switchEvent").item(0).getTextContent());
- map.put("pinchzoomEvent", ele.getElementsByTagName("pinchzoomEvent").item(0).getTextContent());
- map.put("trackballEvent", ele.getElementsByTagName("trackballEvent").item(0).getTextContent());
- map.put("navEvent", ele.getElementsByTagName("navEvent").item(0).getTextContent());
- map.put("majornavEvent", ele.getElementsByTagName("majornavEvent").item(0).getTextContent());
- map.put("syskeysEvent", ele.getElementsByTagName("syskeysEvent").item(0).getTextContent());
- map.put("flipEvent", ele.getElementsByTagName("flipEvent").item(0).getTextContent());
- map.put("anyeventEvent", ele.getElementsByTagName("anyeventEvent").item(0).getTextContent());
- map.put("throttle", ele.getElementsByTagName("throttle").item(0).getTextContent());
- map.put("seed", ele.getElementsByTagName("seed").item(0).getTextContent());
- map.put("times", ele.getElementsByTagName("times").item(0).getTextContent());
- list.add(map);
- //case_list.add(ele.getElementsByTagName("touchEvent").item(0).getTextContent());
- }
- }
- }
return
list;- }
- }
[java] view plain copy
package
MonkeyTool;import
java.util.List;import
javax.swing.JComboBox;- //展示monkey配置項線程
public
class
MonkeyConfigThreadextends
Thread {- String[] s;
- JComboBox monkeyComBox;
- ReadMonkeyConfig config;
- List<String> list;
- String devicesId;
public
MonkeyConfigThread(JComboBox monkeyComBox) {this
.monkeyComBox=monkeyComBox;- }
- @Override
public
void
run() {super
.run();- config=
new
ReadMonkeyConfig(); try
{- list=config.XmlToList_id();
- monkeyComBox.removeAllItems();
if
(list.size()==0){- monkeyComBox.addItem("配置文件中未配置");
- }
else
{for
(String item : list) {- System.out.println(item);
- monkeyComBox.addItem(item);
- }
- }
- }
catch
(Exception e) { - // TODO Auto-generated catch block
- e.printStackTrace();
- }
- }
- }
8.設備面板代碼
[java] view plain copy
package
MonkeyTool;import
java.awt.event.ActionEvent;import
java.awt.event.ActionListener;import
java.util.List;import
javax.swing.JButton;import
javax.swing.JComboBox;import
javax.swing.JLabel;import
javax.swing.JPanel;import
javax.swing.JTextField;public
class
JpanelDevicesextends
JPanel{- AndroidDevicesInfo androidDevicesInfo;
- AndroidPackageInfo androidPackageInfo;
- Object obj;
- String[] s;
- JComboBox devicesComBox;
- JComboBox packageComBox;
- List<String> list;
- String devicesId;
- String devicesId_s;
- JButton jButton;
public
String getDevice(){- //JComboBox<String> devicesComBox=new JComboBox<>();
- //this.devicesComBox=devicesComBox;
return
(String) devicesComBox.getSelectedItem();- }
public
JpanelDevices(){- setBounds(0,15, 720,30);
- setLayout(
null
); - initView();
- addView();
- clickListenser();
- getDevice();
- }
- //初始化控制項
public
void
initView(){- jButton=
new
JButton("刷新設備"); - jButton.setBounds(10,0,100,25);
- devicesComBox=
new
JComboBox(); - packageComBox=
new
JComboBox(); - devicesComBox.setBounds(170, 0,192, 25);
- packageComBox.setBounds(438, 0,265, 25);
- }
public
void
addView(){- add(jButton);
- add(devicesComBox);
- add(packageComBox);
- }
private
void
clickListenser(){- jButton.addActionListener(
new
ActionListener() { - //點擊刷新按鈕,刷新設備Id
- @Override
public
void
actionPerformed(ActionEvent e) {new
DevicesThread(devicesComBox).start();- }
- });
- devicesComBox.addActionListener(
new
ActionListener() { - @Override
public
void
actionPerformed(ActionEvent e) {- // TODO Auto-generated method stub
new
PackageThread(devicesComBox,packageComBox,devicesId).start();- }
- });
- }
- }
[java] view plain copy
package
MonkeyTool;import
java.awt.Color;import
java.awt.event.ActionEvent;import
java.awt.event.ActionListener;import
java.util.HashMap;import
java.util.List;import
javax.swing.BorderFactory;import
javax.swing.JButton;import
javax.swing.JCheckBox;import
javax.swing.JComboBox;import
javax.swing.JLabel;import
javax.swing.JOptionPane;import
javax.swing.JPanel;import
javax.swing.JTextField;public
class
JpanelMonkeyextends
JPanel{- ReadMonkeyConfig config=
new
ReadMonkeyConfig(); - JpanelMonkey jpanelMonkey;
- JLabel touchLable,motionLable,appSwitchLable,throttle,seedLable,timesLable,IgnoreCrashLable,IgnoreTimeoutLable,IgnoreSecurityExceptionLable,KillProcessAfterErrorLable,MonitorNativeCrashLable,pinchzoomLable,trackballLable,navLable,majornavLable,syskeysLable,flipLable,anyeventLable;
- JTextField touchTextField,motionTextField,appswtichTextField,throttleTextField,seedTextField,timesTextField,pinchzoomTextField,trackballTextField,navTextField,majornavTextField,syskeysTextField,flipTextField,anyeventTextField;
- JButton button,button2,monkeyConfigLable,startConfigWrite;
- JCheckBox jCheckBox;
- JCheckBox jCheckBox2;
- JCheckBox jCheckBox3;
- JCheckBox jCheckBox4;
- JCheckBox jCheckBox5;
- JComboBox monkeyComboBox;
- String devicesId_s;
- StringBuilder builder,builderEventPercent;
- String clickPercent,swipePercent,swichPercent,waitTime,seed,times,pinchzoom,trackball,nav,majornav,syskeys,flip,anyevent;
- //CmdUtils cmdUtils=new CmdUtils();
public
JpanelDevices jpanelDevices;- //構造方法穿參實時獲取值
public
JpanelMonkey(JpanelDevices jpanelDevices){- setBorder(BorderFactory.createTitledBorder("分組框")); //設置面板邊框,實現分組框的效果,此句代碼為關鍵代碼
- setBorder(BorderFactory.createLineBorder(Color.blue));
- setLayout(
null
); - setBounds(0,55,720,500);
- initView();
- addView();
this
.jpanelDevices=jpanelDevices;- clickLinstner(jpanelDevices);
new
MonkeyConfigThread(monkeyComboBox).start();- }
- //以下為監聽事件
private
void
clickLinstner(JpanelDevices jpanelDevices) {- //啟動monkey
- button.addActionListener(
new
ActionListener() { public
void
actionPerformed(ActionEvent e) {new
GoMonkey().start();- }
- });
- //停止monkey
- button2.addActionListener(
new
ActionListener() { - @Override
public
void
actionPerformed(ActionEvent e) {- // TODO Auto-generated method stub
new
StopMonkey().start();- }
- });
- //獲取monkey配置項
- monkeyConfigLable.addActionListener(
new
ActionListener() { - @Override
public
void
actionPerformed(ActionEvent e) {- // TODO Auto-generated method stub
new
MonkeyConfigThread(monkeyComboBox).start();- }
- });
- //monkey配置項寫入
- startConfigWrite.addActionListener(
new
ActionListener() { - @Override
public
void
actionPerformed(ActionEvent e) {- // TODO Auto-generated method stub
new
SetMonkeyConfig().start();- }
- });
- }
- //寫入monkey配置項線程
class
SetMonkeyConfigextends
Thread{- @Override
public
void
run() {- // TODO Auto-generated method stub
super
.run();try
{- String id=(String) monkeyComboBox.getSelectedItem();
- List<HashMap<String, String>>monkeyArgs=config.getConfigData(id);
- touchTextField.setText(monkeyArgs.get(0).get("touchEvent"));
- motionTextField.setText(monkeyArgs.get(0).get("motionEvent"));
- appswtichTextField.setText(monkeyArgs.get(0).get("switchEvent"));
- throttleTextField.setText(monkeyArgs.get(0).get("throttle"));
- seedTextField.setText(monkeyArgs.get(0).get("seed"));
- timesTextField.setText(monkeyArgs.get(0).get("times"));
- pinchzoomTextField.setText(monkeyArgs.get(0).get("pinchzoomEvent"));
- trackballTextField.setText(monkeyArgs.get(0).get("trackballEvent"));
- navTextField.setText(monkeyArgs.get(0).get("navEvent"));
- majornavTextField.setText(monkeyArgs.get(0).get("majornavEvent"));
- syskeysTextField.setText(monkeyArgs.get(0).get("syskeysEvent"));
- flipTextField.setText(monkeyArgs.get(0).get("flipEvent"));
- anyeventTextField.setText(monkeyArgs.get(0).get("anyeventEvent"));
- }
catch
(Exception e) { - // TODO Auto-generated catch block
- JOptionPane.showConfirmDialog(
null
, "配置文件不存在","錯誤提示", JOptionPane.DEFAULT_OPTION); - }
- }
- }
class
StopMonkeyextends
Thread{- CmdUtils cmdUtils=
new
CmdUtils(); - @Override
public
void
run() {- // TODO Auto-generated method stub
super
.run();if
((String)jpanelDevices.devicesComBox.getSelectedItem()!=null
){- devicesId_s=" -s "+(String)jpanelDevices.devicesComBox.getSelectedItem();
- }
else
{- devicesId_s=" ";
- }
- //獲取monkey進程並kill
- String commands="adb"+devicesId_s+" shell ps |grep monkey";
- System.out.println(cmdUtils.excuteCmd(commands));
- String log_pid_result=cmdUtils.excuteCmd(commands).get(0);
- System.out.println(log_pid_result);
- String g=log_pid_result.substring(7,15);
- System.out.println(g);
- System.out.println(Thread.currentThread().getName());
- cmdUtils.excuteCmd("adb "+devicesId_s+" shell kill "+g);
- System.out.println("ok");
- }
- }
- //monkey啟動線程
class
GoMonkeyextends
Thread{- CmdUtils cmdUtils=
new
CmdUtils(); - @Override
public
void
run() {- // TODO Auto-generated method stub
super
.run();- String devicesId=(String) jpanelDevices.devicesComBox.getSelectedItem();
- String packageName=(String) jpanelDevices.packageComBox.getSelectedItem();
- builder=
new
StringBuilder(); - builderEventPercent=
new
StringBuilder(); if
(jCheckBox.isSelected()){- builder.append(" --ignore-crashes");
- }
else
{- builder.append("");
- }
if
(jCheckBox2.isSelected()){- builder.append(" --ignore-timeouts");
- }
else
{- builder.append("");
- }
if
(jCheckBox3.isSelected()){- builder.append(" --ignore-security-exceptions");
- }
else
{- builder.append("");
- }
if
(jCheckBox4.isSelected()){- builder.append(" --kill-process-after-error");
- }
else
{- builder.append("");
- }
if
(jCheckBox5.isSelected()){- builder.append(" --monitor-native-crashes");
- }
else
{- builder.append("");
- }
- System.out.println(builder);
if
(touchTextField.getText().equals("")==false
){- clickPercent=" --pct-touch "+touchTextField.getText().toString();
- builderEventPercent.append(clickPercent);
- }
else
{- clickPercent="";
- }
if
(motionTextField.getText().equals("")==false
){- swipePercent=" --pct-motion "+motionTextField.getText().toString();
- builderEventPercent.append(swipePercent);
- }
else
{- swipePercent="";
- }
if
(appswtichTextField.getText().equals("")==false
){- swichPercent=" --pct-appswitch "+appswtichTextField.getText().toString();
- builderEventPercent.append(swichPercent);
- }
else
{- swichPercent="";
- }
if
(throttleTextField.getText().equals("")==false
){- waitTime=" --throttle "+throttleTextField.getText().toString();
- }
else
{- waitTime="";
- }
if
(seedTextField.getText().equals("")==false
){- seed=" -s "+seedTextField.getText().toString();
- }
else
{- seed="";
- }
if
(timesTextField.getText().equals("")){- JOptionPane.showConfirmDialog(
null
, "必須輸入運行次數","輸入提示", JOptionPane.DEFAULT_OPTION); - }
else
{- times=" "+timesTextField.getText().toString();
- }
if
(pinchzoomTextField.getText().equals("")){- pinchzoom="";
- }
else
{- pinchzoom=" --pct-pinchzoom "+pinchzoomTextField.getText().toString();
- builderEventPercent.append(pinchzoom);
- }
if
(trackballTextField.getText().equals("")){- trackball="";
- }
else
{- trackball=" --pct-trackball "+trackballTextField.getText().toString();
- builderEventPercent.append(trackball);
- }
if
(navTextField.getText().equals("")){- nav="";
- }
else
{- nav=" --pct-nav "+navTextField.getText().toString();
- builderEventPercent.append(nav);
- }
if
(majornavTextField.getText().equals("")){- majornav="";
- }
else
{- majornav=" --pct-majornav "+majornavTextField.getText().toString();
- builderEventPercent.append(majornav);
- }
if
(syskeysTextField.getText().equals("")){- syskeys="";
- }
else
{- syskeys=" --pct-syskeys "+syskeysTextField.getText().toString();
- builderEventPercent.append(syskeys);
- }
if
(flipTextField.getText().equals("")){- flip="";
- }
else
{- flip=" --pct-flip "+flipTextField.getText().toString();
- builderEventPercent.append(flip);
- }
if
(anyeventTextField.getText().equals("")){- anyevent="";
- }
else
{- anyevent=" --pct-anyevent "+anyeventTextField.getText().toString();
- builderEventPercent.append(anyevent);
- }
- System.out.println(devicesId);
- System.out.println(packageName);
- //String monkeyCommand="adb -s "+devicesId+" shell monkey -p "+packageName+waitTime+clickPercent+swipePercent+swichPercent+builder.toString()+seedLable+timesLable;
- //System.out.println(j.getSelectedItem().toString());
- String monkeyCommand="adb -s "+devicesId+" shell monkey -p "+packageName+waitTime+builderEventPercent.toString()+builder.toString()+seed+times;
- System.out.println(monkeyCommand);
- System.out.println(cmdUtils.excuteCmd(monkeyCommand));
- }
- }
- //初始化控制項
private
void
initView(){- touchLable=
new
JLabel("Touch Event (%)"); - touchLable.setBounds(10, 30,100, 25);
- motionLable=
new
JLabel("Motion Event (%)"); - motionLable.setBounds(10, 57,100, 25);
- appSwitchLable=
new
JLabel("Appswitch (%)"); - appSwitchLable.setBounds(10, 84,100, 25);
- pinchzoomLable=
new
JLabel("pinchzoom (%)"); - pinchzoomLable.setBounds(10, 111,100, 25);
- trackballLable=
new
JLabel("trackball (%)"); - trackballLable.setBounds(10,138,100, 25);
- navLable=
new
JLabel("nav (%)"); - navLable.setBounds(10,165,100, 25);
- majornavLable=
new
JLabel("majornav (%)"); - majornavLable.setBounds(10,192,100, 25);
- syskeysLable=
new
JLabel("syskeys (%)"); - syskeysLable.setBounds(10,219,100, 25);
- flipLable=
new
JLabel("flip (%)"); - flipLable.setBounds(10,246,100, 25);
- anyeventLable=
new
JLabel("anevent (%)"); - anyeventLable.setBounds(10,273,100, 25);
- throttle=
new
JLabel("Throttle (S)"); - throttle.setBounds(10, 300,100, 25);
- seedLable=
new
JLabel("Seed"); - seedLable.setBounds(10, 327,100, 25);
- timesLable=
new
JLabel("Times"); - timesLable.setBounds(10, 354,100, 25);
- IgnoreCrashLable=
new
JLabel("Ignore Crash"); - IgnoreCrashLable.setBounds(228, 30,120, 25);
- IgnoreTimeoutLable=
new
JLabel("Ignore Timeout"); - IgnoreTimeoutLable.setBounds(228, 57,150, 25);
- IgnoreSecurityExceptionLable=
new
JLabel("Ignore Security Exception"); - IgnoreSecurityExceptionLable.setBounds(228, 84,150, 25);
- KillProcessAfterErrorLable=
new
JLabel("Kill Process After Error"); - KillProcessAfterErrorLable.setBounds(228, 111,150, 25);
- MonitorNativeCrashLable=
new
JLabel("Monitor Native Crash"); - MonitorNativeCrashLable.setBounds(228, 138,150, 25);
- touchTextField=
new
JTextField(); - touchTextField.setBounds(113, 30,80, 25);
- motionTextField=
new
JTextField(); - motionTextField.setBounds(113, 57,80, 25);
- appswtichTextField=
new
JTextField(); - appswtichTextField.setBounds(113, 84,80, 25);
- pinchzoomTextField=
new
JTextField(); - pinchzoomTextField.setBounds(113, 111,80, 25);
- trackballTextField=
new
JTextField(); - trackballTextField.setBounds(113, 138,80, 25);
- navTextField=
new
JTextField(); - navTextField.setBounds(113, 165,80, 25);
- majornavTextField=
new
JTextField(); - majornavTextField.setBounds(113, 192,80, 25);
- syskeysTextField=
new
JTextField(); - syskeysTextField.setBounds(113, 219,80, 25);
- flipTextField=
new
JTextField(); - flipTextField.setBounds(113, 246,80, 25);
- anyeventTextField=
new
JTextField(); - anyeventTextField.setBounds(113, 273,80, 25);
- throttleTextField=
new
JTextField(); - throttleTextField.setBounds(113, 300,80, 25);
- seedTextField=
new
JTextField(); - seedTextField.setBounds(113, 327,80, 25);
- timesTextField=
new
JTextField(); - timesTextField.setBounds(113,354,80, 25);
- jCheckBox=
new
JCheckBox("Ignore Crash"); - jCheckBox.setBounds(202, 30,170, 25);
- jCheckBox2=
new
JCheckBox("Ignore Timeout"); - jCheckBox2.setBounds(202, 57,170, 25);
- jCheckBox3=
new
JCheckBox("Ignore Security Exception"); - jCheckBox3.setBounds(202, 84,170, 25);
- jCheckBox4=
new
JCheckBox("Kill Process After Error"); - jCheckBox4.setBounds(202, 111,170, 25);
- jCheckBox5=
new
JCheckBox("Monitor Native Crash"); - jCheckBox5.setBounds(202, 138,170, 25);
- monkeyConfigLable=
new
JButton("Monkey一鍵配置選擇"); - monkeyConfigLable.setBounds(205,170,170, 25);
- monkeyComboBox=
new
JComboBox<>(); - monkeyComboBox.setBounds(375,170,150, 25);
- startConfigWrite=
new
JButton("配置一鍵寫入"); - startConfigWrite.setBounds(527,170,150, 25);
- button=
new
JButton("Excute Monkey"); - button.setBounds(205, 250,150, 25);
- button2=
new
JButton("Stop Monkey"); - button2.setBounds(360,250,150, 25);
- }
private
void
addView (){- add(touchLable);
- add(motionLable);
- add(appSwitchLable);
- add(throttle);
- add(seedLable);
- add(timesLable);
- add(pinchzoomLable);
- add(trackballLable);
- add(navLable);
- add(majornavLable);
- add(syskeysLable);
- add(flipLable);
- add(anyeventLable);
- add(button);
- add(button2);
- add(touchTextField);
- add(motionTextField);
- add(appswtichTextField);
- add(throttleTextField);
- add(seedTextField);
- add(timesTextField);
- add(pinchzoomTextField);
- add(trackballTextField);
- add(navTextField);
- add(majornavTextField);
- add(syskeysTextField);
- add(flipTextField);
- add(anyeventTextField);
- add(jCheckBox);
- add(jCheckBox2);
- add(jCheckBox3);
- add(jCheckBox4);
- add(jCheckBox5);
- add(monkeyConfigLable);
- add(monkeyComboBox);
- add(startConfigWrite);
- }
- }
10.主類代碼
[java] view plain copy
package
MonkeyTool;import
java.awt.Container;import
javax.swing.JFrame;import
javax.swing.WindowConstants;public
class
MonkeyMainextends
JFrame {public
MonkeyMain(){- setTitle("MonkeyTool");
- setLayout(
null
); - setBounds(0,0,720,500);
- JpanelDevices jpanelDevices=
new
JpanelDevices(); - JpanelMonkey jpanelMonkey=
new
JpanelMonkey(jpanelDevices); - Container container=getContentPane();
- container.add(jpanelDevices);
- container.add(jpanelMonkey);
- setLayout(
null
); - setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
- setVisible(
true
); - }
public
static
void
main(String []args){new
MonkeyMain();- }
- }

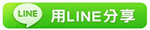
※Tengine 安裝配置全過程
※打開和寫入文件(fopen和fopen s)
TAG:程序員小新人學習 |