Http客戶端工具類
知識
07-16
在平時工作中,我們經常會有請求介面的需求,抽時間總結了一下供大家參考。
依賴包:
- <dependency>
- <groupId>org.apache.httpcomponents</groupId>
- <artifactId>httpclient</artifactId>
- <version>4.5</version>
- </dependency>
工具類文件:
- package com.rz.util;
- import java.io.IOException;
- import java.io.UnsupportedEncodingException;
- import java.net.SocketTimeoutException;
- import java.net.URI;
- import java.net.URISyntaxException;
- import java.net.URL;
- import java.util.ArrayList;
- import java.util.List;
- import java.util.Map;
- import org.apache.commons.httpclient.HttpStatus;
- import org.apache.commons.lang3.StringUtils;
- import org.apache.http.HttpResponse;
- import org.apache.http.NameValuePair;
- import org.apache.http.ParseException;
- import org.apache.http.client.config.RequestConfig;
- import org.apache.http.client.entity.UrlEncodedFormEntity;
- import org.apache.http.client.methods.CloseableHttpResponse;
- import org.apache.http.client.methods.HttpDelete;
- import org.apache.http.client.methods.HttpGet;
- import org.apache.http.client.methods.HttpPost;
- import org.apache.http.client.methods.HttpPut;
- import org.apache.http.client.utils.URLEncodedUtils;
- import org.apache.http.entity.StringEntity;
- import org.apache.http.impl.client.CloseableHttpClient;
- import org.apache.http.impl.client.HttpClients;
- import org.apache.http.message.BasicNameValuePair;
- import org.apache.http.util.EntityUtils;
- import org.slf4j.Logger;
- import org.slf4j.LoggerFactory;
- import com.alibaba.fastjson.JSONObject;
- /**
- * HTTP客戶端幫助類
- */
- public class HttpClientUtil {
- private static final Logger LOG = LoggerFactory.getLogger(HttpClientUtil.class);
- public static final String CHAR_SET = "UTF-8";
- private static CloseableHttpClient httpClient;
- private static int socketTimeout = 30000;
- private static int connectTimeout = 30000;
- private static int connectionRequestTimeout = 30000;
- // 配置總體最大連接池(maxConnTotal)和單個路由連接最大數(maxConnPerRoute),默認是(20,2)
- private static int maxConnTotal = 200; // 最大不要超過1000
- private static int maxConnPerRoute = 100;// 實際的單個連接池大小,如tps定為50,那就配置50
- static {
- RequestConfig config = RequestConfig.custom()
- .setSocketTimeout(socketTimeout)
- .setConnectTimeout(connectTimeout)
- .setConnectionRequestTimeout(connectionRequestTimeout).build();
- httpClient = HttpClients.custom().setDefaultRequestConfig(config)
- .setMaxConnTotal(maxConnTotal)
- .setMaxConnPerRoute(maxConnPerRoute).build();
- }
- public static CloseableHttpClient getClient() {
- return httpClient;
- }
- public static String get(String url) {
- try {
- return get(url, null, null);
- } catch (IOException e) {
- LOG.error("HttpClientUtil.get()報錯:", e);
- }
- return null;
- }
- public static String get(String url, Map<String, String> map) {
- try {
- return get(url, map, null);
- } catch (IOException e) {
- LOG.error("HttpClientUtil.get()報錯:", e);
- }
- return null;
- }
- public static String get(String url, String charset) {
- try {
- return get(url, null, charset);
- } catch (IOException e) {
- LOG.error("HttpClientUtil.get()報錯:", e);
- }
- return null;
- }
- public static String get(String url, Map<String, String> paramsMap, String charset) throws IOException {
- if ( StringUtils.isBlank(url) ) {
- return null;
- }
- charset = (charset == null ? CHAR_SET : charset);
- if (null != paramsMap && !paramsMap.isEmpty()) {
- List<NameValuePair> params = new ArrayList<NameValuePair>();
- for (Map.Entry<String, String> map : paramsMap.entrySet()) {
- params.add(new BasicNameValuePair(map.getKey(), map.getValue()));
- }
- //GET方式URL參數編碼
- String querystring = URLEncodedUtils.format(params, charset);
- if(StringUtils.contains(url, "?")) {
- url += "&" + querystring;
- } else {
- url += "?" + querystring;
- }
- }
- HttpGet httpGet = new HttpGet(url);
- httpGet.addHeader("Accept-Encoding", "*");
- CloseableHttpResponse response = null;
- try {
- response = getClient().execute(httpGet);
- } catch (SocketTimeoutException e) {
- LOG.error("請求超時,1秒後將重試1次:"+ url, e);
- try {
- Thread.sleep(1000);
- } catch (InterruptedException e1) { }
- response = getClient().execute(httpGet);
- }
- // 狀態不為200的異常處理。
- return EntityUtils.toString(response.getEntity(), charset);
- }
- /**
- * 提供返回json結果的get請求(ESG專用)
- * @param url
- * @param charset
- * @return
- * @throws IOException */
- public static String getResponseJson(String url, Map<String, String> map) {
- try {
- return getResponseJson(url, map, null);
- } catch (IOException e) {
- LOG.error("HttpClientUtil.getResponseJson()報錯:", e);
- }
- return null;
- }
- public static String getResponseJson(String url, Map<String, String> paramsMap, String charset) throws IOException {
- if ( StringUtils.isBlank(url) ) {
- return null;
- }
- charset = (charset == null ? CHAR_SET : charset);
- if (null != paramsMap && !paramsMap.isEmpty()) {
- List<NameValuePair> params = new ArrayList<NameValuePair>();
- for (Map.Entry<String, String> map : paramsMap.entrySet()) {
- params.add(new BasicNameValuePair(map.getKey(), map.getValue()));
- }
- //GET方式URL參數編碼
- String querystring = URLEncodedUtils.format(params, charset);
- if(StringUtils.contains(url, "?")) {
- url += "&" + querystring;
- } else {
- url += "?" + querystring;
- }
- }
- HttpGet httpGet = new HttpGet(url);
- httpGet.addHeader("Accept-Encoding", "*");
- httpGet.addHeader("Accept", "application/json;charset=UTF-8");
- CloseableHttpResponse response = null;
- try {
- response = getClient().execute(httpGet);
- } catch (SocketTimeoutException e) {
- // LOG.error("請求超時,1秒後將重試1次:"+ url, e);
- LOG.error(e.getMessage()+ "請求超時,1秒後將重試1次:"+ url);
- try {
- Thread.sleep(1000);
- } catch (InterruptedException e1) { }
- response = getClient().execute(httpGet);
- }
- // 狀態不為200的異常處理。
- return EntityUtils.toString(response.getEntity(), charset);
- }
- public static String delete(String url, Map<String, String> paramsMap, String charset) throws IOException {
- if ( StringUtils.isBlank(url) ) {
- return null;
- }
- charset = (charset == null ? CHAR_SET : charset);
- if (null != paramsMap && !paramsMap.isEmpty()) {
- List<NameValuePair> params = new ArrayList<NameValuePair>();
- for (Map.Entry<String, String> map : paramsMap.entrySet()) {
- params.add(new BasicNameValuePair(map.getKey(), map.getValue()));
- }
- //GET方式URL參數編碼
- String querystring = URLEncodedUtils.format(params, charset);
- if(StringUtils.contains(url, "?")) {
- url += "&" + querystring;
- } else {
- url += "?" + querystring;
- }
- }
- HttpDelete httpDelete = new HttpDelete(url);
- httpDelete.addHeader("Accept-Encoding", "*");
- CloseableHttpResponse response = getClient().execute(httpDelete);
- // 狀態不為200的異常處理。
- return EntityUtils.toString(response.getEntity(), charset);
- }
- public static String post(String url, String request) {
- return post(url, request, null);
- }
- public static String post(String url, String request, String charset) {
- if ( StringUtils.isBlank(url) ) {
- return null;
- }
- String res = null;
- CloseableHttpResponse response = null;
- try {
- charset = (charset == null ? CHAR_SET : charset);
- StringEntity entity = new StringEntity(request, charset);
- HttpPost httpPost = new HttpPost(url);
- httpPost.addHeader("Content-Type", "application/json;charset=utf-8");
- httpPost.addHeader("Accept-Encoding", "*");
- httpPost.setEntity(entity);
- try {
- response = getClient().execute(httpPost);
- } catch (SocketTimeoutException e) {
- LOG.error("請求超時,1秒後將重試1次:"+ url, e);
- try {
- Thread.sleep(1000);
- } catch (InterruptedException e1) { }
- response = getClient().execute(httpPost);
- }
- res = EntityUtils.toString(response.getEntity());
- // 狀態不為200的異常處理。
- } catch (ParseException e) {
- LOG.error("HTTP Post(), ParseException: ", e);
- } catch (IOException e) {
- LOG.error("HTTP Post(), IOException: ", e);
- } finally {
- if (response != null) {
- try {
- response.close();
- } catch (IOException e) {
- }
- }
- }
- return res;
- }
- public static String post(String url, Map<String, String> map) {
- return post(url, map, null);
- }
- public static String post(String url, Map<String, String> paramsMap, String charset) {
- if ( StringUtils.isBlank(url) ) {
- return null;
- }
- String res = null;
- CloseableHttpResponse response = null;
- try {
- charset = (charset == null ? CHAR_SET : charset);
- List<NameValuePair> params = new ArrayList<NameValuePair>();
- for (Map.Entry<String, String> map : paramsMap.entrySet()) {
- params.add(new BasicNameValuePair(map.getKey(), map.getValue()));
- }
- UrlEncodedFormEntity formEntity = new UrlEncodedFormEntity(params, charset);
- HttpPost httpPost = new HttpPost(url);
- httpPost.addHeader("Accept-Encoding", "*");
- httpPost.setEntity(formEntity);
- try {
- response = getClient().execute(httpPost);
- } catch (SocketTimeoutException e) {
- LOG.error("請求超時,1秒後將重試1次:"+ url, e);
- try {
- Thread.sleep(1000);
- } catch (InterruptedException e1) { }
- response = getClient().execute(httpPost);
- }
- res = EntityUtils.toString(response.getEntity());
- // 狀態不為200的異常處理。
- if (response.getStatusLine().getStatusCode() != 200) {
- throw new IOException(res);
- }
- } catch (IOException e) {
- LOG.error("HTTP Post(), IOException: ", e);
- } finally {
- if (response != null) {
- try {
- response.close();
- } catch (IOException e) {
- }
- }
- }
- return res;
- }
- /**
- * 提供返回json結果的post請求
- * @param url
- * @param map
- * @param charset
- * @return json
- * @throws IOException */
- public static String postResponseJson(String url, Map<String, String> map) {
- return postResponseJson(url, map, null);
- }
- /**
- * Put方式提交
- * @param url
- * @param paramsMap
- * @param charset
- * @return response.getEntity() */
- public static String postResponseJson(String url, Map<String, String> paramsMap, String charset) {
- if ( StringUtils.isBlank(url) ) {
- return null;
- }
- String res = null;
- CloseableHttpResponse response = null;
- try {
- charset = (charset == null ? CHAR_SET : charset);
- List<NameValuePair> params = new ArrayList<NameValuePair>();
- for (Map.Entry<String, String> map : paramsMap.entrySet()) {
- params.add(new BasicNameValuePair(map.getKey(), map.getValue()));
- }
- UrlEncodedFormEntity formEntity = new UrlEncodedFormEntity(params, charset);
- //解決url中的一些特殊字元
- URI uri = null;
- HttpPost httpPost = null;
- try {
- URL url2 = new URL(url);
- uri = new URI(url2.getProtocol(), url2.getUserInfo(), url2.getHost(), url2.getPort(), url2.getPath(), url2.getQuery(), null);
- } catch (URISyntaxException e) {
- LOG.error("URL中存在非法編碼:"+ url, e);
- uri = null;
- }
- if(uri != null) {
- httpPost = new HttpPost( uri );
- } else {
- httpPost = new HttpPost( url );
- }
- httpPost.addHeader("Accept-Encoding", "*");
- httpPost.addHeader("Accept", "application/json;charset=UTF-8");
- httpPost.setEntity(formEntity);
- try {
- response = getClient().execute(httpPost);
- } catch (SocketTimeoutException e) {
- // LOG.error("請求超時,1秒後將重試1次:"+ url, e);
- LOG.error(e.getMessage()+ "請求超時,1秒後將重試1次:"+ url);
- try {
- Thread.sleep(1000);
- } catch (InterruptedException e1) { }
- response = getClient().execute(httpPost);
- }
- res = EntityUtils.toString(response.getEntity());
- // 狀態不為200的異常處理。
- if (response.getStatusLine().getStatusCode() != 200) {
- throw new IOException(res);
- }
- } catch (IOException e) {
- LOG.error("postResponseJson方法報錯: ", e);
- } finally {
- if (response != null) {
- try {
- response.close();
- } catch (IOException e) {
- }
- }
- }
- return res;
- }
- /**
- * Put方式提交
- * @param url
- * @param paramsMap
- * @param charset
- * @return response.getEntity() */
- public static String put(String url, Map<String, String> paramsMap, String charset) {
- if ( StringUtils.isBlank(url) ) {
- return null;
- }
- String res = null;
- CloseableHttpResponse response = null;
- try {
- charset = (charset == null ? CHAR_SET : charset);
- List<NameValuePair> params = new ArrayList<NameValuePair>();
- for (Map.Entry<String, String> map : paramsMap.entrySet()) {
- params.add(new BasicNameValuePair(map.getKey(), map.getValue()));
- }
- UrlEncodedFormEntity formEntity = new UrlEncodedFormEntity(params, charset);
- HttpPut httpPut = new HttpPut(url);
- httpPut.addHeader("Accept-Encoding", "*");
- httpPut.setEntity(formEntity);
- try {
- response = getClient().execute(httpPut);
- } catch (SocketTimeoutException e) {
- LOG.error("請求超時,1秒後將重試1次:"+ url, e);
- try {
- Thread.sleep(1000);
- } catch (InterruptedException e1) { }
- response = getClient().execute(httpPut);
- }
- res = EntityUtils.toString(response.getEntity());
- // 狀態不為200的異常處理。
- if (response.getStatusLine().getStatusCode() != 200) {
- throw new IOException(res);
- }
- } catch (IOException e) {
- LOG.error("HTTP put(), IOException: ", e);
- } finally {
- if (response != null) {
- try {
- response.close();
- } catch (IOException e) {
- }
- }
- }
- return res;
- }
- /**
- * Titel 提交HTTP請求,獲得響應(application/x-www-form-urlencoded) Description
- * 使用NameValuePair封裝參數,適用於下載文件。
- * @param serviceURI : 介面地址
- * @param timeOut : 超時時間
- * @param params : 請求參數
- * @param charset : 參數編碼
- * @return CloseableHttpResponse */
- public static CloseableHttpResponse submitPostHttpReq(final String serviceURI, final int timeOut,
- final Map<String, String> pmap, final String charset) {
- CloseableHttpResponse response = null;
- LOG.info("即將發起HTTP請求!serviceURI:" + serviceURI);
- // 遍歷封裝參數
- List<NameValuePair> formParams = new ArrayList<NameValuePair>();
- for (String key : pmap.keySet()) {
- NameValuePair pair = new BasicNameValuePair(key, pmap.get(key));
- formParams.add(pair);
- }
- // 轉換為from Entity
- UrlEncodedFormEntity fromEntity = null;
- try {
- fromEntity = new UrlEncodedFormEntity(formParams, CHAR_SET);
- } catch (UnsupportedEncodingException e) {
- LOG.error("創建UrlEncodedFormEntity異常,編碼問題: " + e.getMessage());
- return null;
- }
- // 創建http post請求
- HttpPost httpPost = new HttpPost(serviceURI);
- httpPost.setEntity(fromEntity);
- // 設置請求和傳輸超時時間
- RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(timeOut).setConnectTimeout(timeOut).build();
- httpPost.setConfig(requestConfig);
- // 提交請求、獲取響應
- LOG.info("提交http請求!serviceURI:" + serviceURI);
- CloseableHttpClient httpclient = HttpClients.createDefault();
- try {
- response = httpclient.execute(httpPost);
- } catch (SocketTimeoutException e) {
- LOG.error("請求超時,1秒後將重試1次:"+ serviceURI, e);
- try {
- Thread.sleep(1000);
- response = httpclient.execute(httpPost);
- } catch (Exception e1) {
- LOG.error("超時後再次請求報錯:", e);
- response = null;
- }
- } catch (IOException e) {
- LOG.error("httpclient.execute異常!" + e.getMessage());
- response = null;
- }
- LOG.info("提交http請求!serviceURI:" + serviceURI);
- return response;
- }
- public static int getSocketTimeout() {
- return socketTimeout;
- }
- public static void setSocketTimeout(int socketTimeout) {
- HttpClientUtil.socketTimeout = socketTimeout;
- }
- public static int getConnectTimeout() {
- return connectTimeout;
- }
- public static void setConnectTimeout(int connectTimeout) {
- HttpClientUtil.connectTimeout = connectTimeout;
- }
- public static int getConnectionRequestTimeout() {
- return connectionRequestTimeout;
- }
- public static void setConnectionRequestTimeout(int connectionRequestTimeout) {
- HttpClientUtil.connectionRequestTimeout = connectionRequestTimeout;
- }
- /**
- * 請求josn串,返回josn對象
- * */
- public static JSONObject doPost(String url, String jsonStr) {
- HttpPost post = new HttpPost(url);
- JSONObject response = null;
- try {
- StringEntity s = new StringEntity(jsonStr,"UTF-8");
- s.setContentType("application/json");// 發送json數據需要設置contentType
- post.setEntity(s);
- HttpResponse res = getClient().execute(post);
- if (res.getStatusLine().getStatusCode() == HttpStatus.SC_OK) {
- String result = EntityUtils.toString(res.getEntity());// 返回json格式:
- response = JSONObject.parseObject(result);
- }
- } catch (Exception e) {
- throw new RuntimeException(e);
- }
- return response;
- }
- }
完

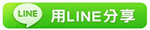
※應用程序無法正常啟動0xc0150002解決方案
※win7下tomcat8的配置
TAG:程序員小新人學習 |