C++流類庫繼承體系(IO流,文件流,串流)和 字元串流的基本操作
一、IO、流
數據的輸入和輸出(input/output簡寫為I/O)
對標準輸入設備和標準輸出設備的輸入輸出簡稱為標準I/O
對在外存磁碟上文件的輸入輸出簡稱為文件I/O
對內存中指定的字元串存儲空間的輸入輸出簡稱為串I/O
數據輸入輸出的過程,可以形象地看成流
從流中獲取數據的操作稱為「提取」(輸入)操作
向流中添加數據的操作稱為「插入」(輸出)操作
標準輸入輸出流
文件流
字元串流
二、流類庫繼承體系、四個輸入輸出對象
流庫具有兩個平行的基類:streambuf 和 ios 類,所有流類均以兩者之一作為基類
streambuf 類提供對緩衝區的低級操作:設置緩衝區、對緩衝區指針操作區存/取字元
ios_base、ios 類記錄流狀態,支持對streambuf 的緩衝區輸入/輸出的格式化或非格式化轉換
stringbuf:使用串保存字元序列。擴展 streambuf 在緩衝區提取和插入的管理
filebuf:使用文件保存字元序列。包括打開文件;讀/寫、查找字元
如下圖:
C++為用戶進行標準I/O操作定義了四個類對象: cin,cout,cerr和clog
cin為istream流類的對象,代表標準輸入設備鍵盤,後三個為ostream流類的對象
cout代表標準輸出設備顯示器
cerr和clog含義相同,均代表錯誤信息輸出設備顯示器
三、ostream流 的操作,istream 流的操作
(一)、ostream流 的操作:
1、operator <<
<<操作返回一個ostream對象的引用,所以可以連續使用
2、put( )
輸出單個字元
返回一個ostream對象的引用
cout.put(『H』).put(『i』);
3、write( )
write(buf, len)
write( )返回一個ostream對象的引用
cout.write (buf, len) //char buf[len]
C++ Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
#include <iostream>
using namespace std;
int main(void)
{
int n = 100;
int n2 = 200;
cout << n << " " << n2 << endl;
cout.put("H");
cout.put("i");
cout.put(" ");
cout.put("H").put("i").put("
");
char buf[] = "test!!!!!";
cout.write(buf, 5);
return 0;
}
(二)、istream流 的操作:
1、opeartor>>操作
<<操作返回一個ostream對象的引用,所以可以連續使用
2、get( )
get( )操作:
讀取單個字元
返回一個整數
字元的ASCII碼
get(char&)操作:
讀取單個字元
返回一個istream對象的引用
3、getline( )
讀取一行
遇到回車鍵
返回istream對象的引用
getline()操作與>>的區別:
char string1 [256],
cin.getline(string1, 256); //get a whole line, 以" "結尾
cin >> string1; //stop at the 1st blank space
4、read( )
read(buf, len)
返回一個istream對象的引用
對空白字元(包括"
")照讀不誤
5、peek( ) 與 putpack()
peek:查看而不讀取
putback:將一個字元添加到流
C++ Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
#include <iostream>
using namespace std;
int main(void)
{
//int n;
//char ch;
//cin>>n>>ch;
//cout<<"n="<<n<<" "<<"ch="<<ch<<endl;
//int ch = cin.get();
//cout<<ch<<endl;
//char ch1;
//char ch2;
//cin.get(ch1).get(ch2);
//cout<<ch1<<" "<<ch2<<endl;
char buf[10] = {0};
cin.getline(buf, 10);
cout << buf << endl;
//char buf[10] = {0};
//cin>>buf;
//cout<<buf<<endl;
//char buf[10] = {0};
//cin.read(buf, 5);
//cout<<buf<<endl;
/*char c[10], c2, c3;
c2 = cin.get( );
c3 = cin.get( );
cin.putback( c2 );
cin.getline( &c[0], 10);
cout << c << endl;*/
return 0;
}
二、字元串流的基本操作
istringstream,由istream派生而來,提供讀string的功能
ostringstream,由ostream派生而來,提供寫string的功能
stringstream,由iostream派生而來,提供讀寫string的功能
(一)、分割單詞
C++ Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
#include <iostream>
#include <sstream>
using namespace std;
int main(void)
{
string line;
string word;
while (getline(cin, line))
{
istringstream iss(line);
while (iss >> word)
cout << word << "#";
cout << endl;
}
return 0;
}
(二)、字元串與double 類型互相轉換
C++ Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
#include <iostream>
#include <sstream>
using namespace std;
string doubletostr(double val)
{
ostringstream oss;
oss << val;
return oss.str(); // return string copy of character array
}
double strtodouble(const string &str)
{
istringstream iss(str);
double val;
iss >> val;
return val;
}
int main(void)
{
double val = 55.55;
string str = doubletostr(val);
cout << str << endl;
str = "123.123";
val = strtodouble(str);
cout << val << endl;
return 0;
}
(三)、實現類似sscanf, sprinft 的功能
C++ Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
#include <iostream>
#include <sstream>
using namespace std;
int main(void)
{
//192,168,0,100;
//sscanf,sprintf;
//istringstream iss("192,168,0,100");
//int v1;
//int v2;
//int v3;
//int v4;
//char ch;
//iss>>v1>>ch>>v2>>ch>>v3>>ch>>v4;
//ch = ".";
//ostringstream oss;
//oss<<v1<<ch<<v2<<ch<<v3<<ch<<v4;
//cout<<oss.str()<<endl;
string buf("192,168,0,100");
stringstream ss(buf);
int v1;
int v2;
int v3;
int v4;
char ch;
ss >> v1 >> ch >> v2 >> ch >> v3 >> ch >> v4;
ch = ".";
stringstream ss2;
ss2 << v1 << ch << v2 << ch << v3 << ch << v4;
cout << ss2.str() << endl;
return 0;
}
輸出為192.168.0.100

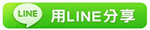
※spring整體架構
※如何在伺服器里刪除用戶,添加用戶,更改用戶密碼
TAG:程序員小新人學習 |