List順序表,鏈表隊列,棧,字典
基礎知識
順序表就是一個數組!!! 只不過數組是被限制的 順序表是內存地址連續的, 順序表方便查詢 追加 遍歷 不適合做刪除和插入 因為會頻繁的進行賦值操作
順序表有長度限制
鏈表 頭插法,逆序,尾插法,正序
鏈表是由一個個Node組成的, Node裡面有兩個元素 data Node的下一個地址
當Node的.Next為Null的時候鏈表到尾部 鏈表是沒有長度限制的 鏈表要時刻注意別斷鏈
增加鏈表和刪除鏈表都得找到誰? 要操作的前一個元素 鏈表在筆試中佔有很大比例 鏈表簡單比劃 鏈表分為兩種{帶頭鏈表和不帶頭的鏈表 一般都採用帶頭的 方便管理 } 若將一個數據逆置就採用在頭部插入的方式 exp index = 0
若要鏈表數據和輸入數據一致就在用尾部插入法 exp index = length
順序表更省空間,鏈表浪費空間(更好的利用空間)
棧和隊列都是特殊的線性表(受限線性表)
順序棧 順序隊列 原型是順表
鏈棧 鏈隊列 原型就是鏈表
順序表
MyList類
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MyList
{
//泛型
class MySeqList<T>
{
private int _flag;//實際個數
private T[] _ints;//儲存的空間,類似數組
public MySeqList()//構造函數,賦初值
{
_ints = new T[30];
_flag = 0;
}
public MySeqList(int count)//重載
{
_ints = new T[count];
_flag = 0;
}
//添加
public void AddItem(T Item)
{
if (_flag >= _ints.Length)
{
Console.WriteLine("空間溢出");
return;
}
_ints[_flag] = Item;
_flag++;
}
//由下標刪除
public T RemoveAt(int index)
{
T returnValue = default(T);
if (index<0||index>=_flag)
{
Console.WriteLine("索引出界");
goto returnTip;
}
returnValue = _ints[index];
for (int i = index; i < _flag-1; i++)
{
_ints[i] = _ints[i + 1];
}
_flag--;
returnTip:
return returnValue;
}
//由數值刪除,未找到返回-1
public void Remove(T removeItem)
{
int tmpIndex = -1;
for (int i = 0; i < _flag; i++)
{
if (_ints[i].Equals(removeItem))
{
tmpIndex = i;
break;
}
}
if (tmpIndex != -1)
{
RemoveAt(tmpIndex);
}
}
//查找
public int IndexOf(T Item)
{
int returnValue = -1;
for (int i = 0; i < _flag; i++)
{
if (_ints[i].Equals(Item))
{
returnValue = i;
break;
}
}
return returnValue;
}
//插入
public void Insert(int index,T Item)
{
if (_flag>=_ints.Length)
{
Console.WriteLine("溢出");
return;
}
if (index > _flag || index < 0)
{
Console.WriteLine("索引出界");
return;
}
for (int i = _flag; i >index; i--)
{
_ints[i] = _ints[i - 1];
_ints[index] = Item;
_flag++;
}
}
//遍歷
public void ShowItem(Action<T> ac)
{
for (int i = 0; i < _flag; i++)
{
ac(_ints[i]);
}
}
//清除所有
public void Clear()
{
_flag = 0;
}
//逆序
public void Reverse()
{
T n;
for (int i = 0; i < _flag/2; i++)
{
n = _ints[i];
_ints[i] = _ints[_flag - 1-i];
_ints[_flag - 1-i] = n;
}
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
主類
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MyList
{
class MyClass
{
public string Name;
public MyClass(int i)
{
Name = "zhangsan " + i;
}
}
class Program
{
static void show(MyClass my)
{
Console.WriteLine(my.Name);
}
static void Main(string[] args)
{
MySeqList<MyClass> intList = new MySeqList<MyClass>();
intList.AddItem(new MyClass(1));
intList.AddItem(new MyClass(2));
intList.AddItem(new MyClass(3));
intList.AddItem(new MyClass(4));
intList.AddItem(new MyClass(5));
intList.ShowItem(show);
intList.Reverse();
intList.ShowItem(show);
//intList.ShowItem(show);
Console.ReadLine();
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
鏈表
LinkList類
using System;
namespace 鏈表
{
//一般鏈表都是有頭部節點的 簡稱為頭結點 頭結點不參與運算
public class LinkList
{
private Node _head;
private int _count;
public LinkList()
{
//new 對象 _head.next --> null head.data = 0
_head = new Node();
_count = 0;
}
public void AddItem(Node newNode)
{
//找到頭結點
Node tmpNode = _head;
//循環找到最後結點
while (tmpNode.Next != null)
{
//一直下移
tmpNode = tmpNode.Next;
}
//將最後結點和即將插入的結點鏈接
tmpNode.Next = newNode;
//個數++
_count++;
}
public int GetLength()
{
return _count;
}
public void Insert(int index, Node newNode)
{
//0
if (index < 0 || index > _count)
{
Console.WriteLine("Over");
return;
}
Node tmpNode = _head;
for (int i = 0; i < index; i++)
{
tmpNode = tmpNode.Next;
}
//tmpNode? index的前一個結點
newNode.Next = tmpNode.Next;
tmpNode.Next = newNode;
_count++;
//0~l-1
// l
}
/// <summary>
/// 第一個就是index 第二個是value
/// </summary>
/// <param name="ac"></param>
public void ShowItem(Action<int,int> ac)
{
if (_count == 0)
{
Console.WriteLine("空");
return;
}
Node tmNode = _head.Next;
for (int i = 0; i < _count; i++)
{
ac(i, tmNode.Data);
//下移動
tmNode = tmNode.Next;
}
}
public int RemoveAt(int index)
{
//定義一個data返回值
int returnValue = default(int);
//判斷是否出界
if (index < 0 || index >=_count)
{
Console.WriteLine("error");
goto returnTip;
}
//刪除結點的前一個結點
Node tmpNode = _head;
//循環走
for (int i = 0; i < index; i++)
{
tmpNode = tmpNode.Next;
}
//要刪除的結點
Node deleteNode = tmpNode.Next;
//牽手刪除結點的後一個結點
tmpNode.Next = tmpNode.Next.Next;
//不讓其連接
deleteNode.Next = null;
//個數--
_count--;
//返回刪除結點的數據data
returnValue = deleteNode.Data;
returnTip:
return returnValue;
}
public void Clear()
{
_head.Next = null;
_count = 0;
}
public void Reverse()
{
Node node = _head.Next;
Node temp = node;
while (temp != null)//不只有頭結點
{
node = _head.Next;
if (temp != null)
{
_head.Next = temp;
temp = temp.Next;
_head.Next.Next = node;
}
else
{
temp = temp.Next;
node.Next.Next = null;
}
}
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
Node類
namespace 鏈表
{
public class Node
{
public int Data;
//這個就是地址
public Node Next;
/// <summary>
/// 構造函數目的就是初始化
/// </summary>
public Node()
{
Data = default(int);
Next = null;
}
public Node(int value)
{
Data = value;
Next = null;
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
主類
using System;
namespace 鏈表
{
internal class Program
{
public static void Show(int index, int value)
{
Console.WriteLine("第{0}個元素是{1}",index+1,value);
}
public static void Main(string[] args)
{
LinkList linkList = new LinkList();
linkList.AddItem(new Node(1));
linkList.AddItem(new Node(2));
linkList.AddItem(new Node(3));
linkList.AddItem(new Node(4));
linkList.AddItem(new Node(5));
linkList.Insert(1,new Node(1000));
//1 1000 2 3 4 5
linkList.Clear();
Console.WriteLine(linkList.RemoveAt(1));
Console.WriteLine(linkList.GetLength());
linkList.ShowItem(Show);
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
棧,隊列
棧:
壓棧: push
出棧: pop
獲取棧頂: peek
判斷有沒有:cont***
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 棧
{
class Program
{
static void Main(string[] args)
{
Stack<int> stack = new Stack<int>();
//照片人臉識別
stack.Push(10);
stack.Push(20);
stack.Push(30);
stack.Push(40);
stack.Push(50);
stack.Push(60);
stack.Push(70);
stack.Push(80);
stack.Push(90);
stack.Push(100);
stack.Push(110);
//Console.WriteLine(stack.Pop());
;
//遍歷棧
int[] ints= stack.ToArray();
Console.WriteLine(ints[0]);
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
隊列:
入隊:
出隊:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 隊列
{
class Program
{
static void Main(string[] args)
{
List<int> list = new List<int>();
Queue<int> queue = new Queue<int>();
queue.Enqueue(10);
queue.Enqueue(20);
queue.Enqueue(30);
queue.Enqueue(40);
queue.Enqueue(50);
queue.Enqueue(60);
queue.Enqueue(70);
Console.WriteLine(queue.Dequeue());
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
字典
遍歷字典的方法
(1)KeyValuePair
foreach (KeyValuePair<string, int> pair in dic)
(2)Dictionary.Values
foreach (int value in dic.Values)
(3)Dictionary.Keys
foreach (string key in dic.Keys)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 字典
{
class Program
{
static void Main(string[] args)
{
// //string string 固定格式
// //name age
// //fenshuA fenshuB
// //GameObj Comm
// Dictionary<string,string> userDictionary = new Dictionary<string, string>();
// userDictionary.Add("zhangsan","1324");
// //userDictionary.Add("zhangsan","1324");
//// Console.WriteLine(userDictionary.Remove("lixi"));
// //userDictionary.ContainsKey()
// ;
// foreach (var v in userDictionary)
// {
// Console.WriteLine(v.Key);
// Console.WriteLine(v.Value);
// }
// Console.WriteLine("=========================================");
// userDictionary["zhangsan"] = "zhangsangfeng";
// Console.WriteLine(userDictionary["zhangsan"]);
// //foreach (var v in userDictionary)
// //{
// // Console.WriteLine(v.Key);
// // Console.WriteLine(v.Value);
// //}
//是一種類型固定的可變長數組!!!
List<int> list = new List<int>();
list.Add(10);//添加到末尾
list.AddRange(new int[]{1,2,3,4,5,6,7,8,9,0}); // 添加一個數組
list.Remove(10000); //刪除該值
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
快速學習外語的方法!
攝影/後期:進了森林迷了璐
場地:八里湖破蘆葦地
終於為那一身江南煙雨覆了天下,容華謝後,不過一場

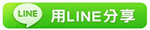
※快速自定義Cordova插件(-配置文件)
※tensorflow隨筆-tf.nn.conv2d卷積運算
TAG:程序員小新人學習 |