實現文件上傳,以及表單提交成功的回調函數
最近在項目中需要實現圖片的上傳,並且成功後返回圖片上傳保存路徑,通過查找資料探索研究,實現了項目功能需求,記在這方便自己以後查閱,也為有同樣需求的碼友分享,功能實現比較簡單,如果有好的建議和實現方法,還望多多指教。
下載地址:http://download.csdn.net/detail/xiaole0313/8984223
主要將實現一下兩個功能:
1、使用commons-fileupload實現文件的上傳(包括圖片);
2、使用jquery-form.js實現表單提交成功的回調函數。
頁面設計fileupload.jsp:
Html代碼
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>文件上傳</title>
<script src="static/js/jquery.min.js" type="text/javascript"></script>
<script type="text/javascript" src="static/js/jquery-form.js"></script>
<script type="text/javascript">
function subimtBtn() {
var form = $("form[name=fileForm]");
var options = {
url:"${pageContext.servletContext.contextPath}/servlet/imageUploadServlet",
type:"post",
success:function(data)
{
var jsondata = eval("("+data+")");
if(jsondata.error == "0"){
var url = jsondata.url;
alert(url)
$("#img").attr("src",url);
}else{
var message = jsondata.message;
alert(message);
}
}
};
form.ajaxSubmit(options);
//$("#fileForm").submit();
}
</script>
</head>
<body>
<div class="modal-body">
<form action="${pageContext.servletContext.contextPath}/servlet/imageUploadServlet" enctype="multipart/form-data" method="post" id="fileForm" name="fileForm">
<input type="file" name="filename">
</form>
</div>
<div class="modal-footer">
<button class="btn btn-primary" onclick="subimtBtn();">提交</button>
</div>
<div>
<img alt="img" id="img">
</div>
</body>
</html>
servlet實現:imageUploadServlet.java
Java代碼
package com.system.comtrol;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import java.text.SimpleDateFormat;
import java.util.Arrays;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import net.sf.json.JSONObject;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.FileItemFactory;
import org.apache.commons.fileupload.FileUploadException;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
/**
* Servlet implementation class ImageUploadServlet
*/
@WebServlet("/servlet/imageUploadServlet")
public class ImageUploadServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#service(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
PrintWriter out = response.getWriter();
// 文件保存目錄路徑
String savePath = request.getServletContext().getRealPath("/")+ "attached";
// 文件保存目錄URL
String saveUrl = request.getContextPath() + "/attached";
// 定義允許上傳的文件擴展名
HashMap<String, String> extMap = new HashMap<String, String>();
extMap.put("image", "gif,jpg,jpeg,png,bmp");
// 最大文件大小
long maxSize = 1000000;
response.setContentType("text/html; charset=UTF-8");
if (!ServletFileUpload.isMultipartContent(request)) {
out.println(getError("請選擇文件。"));
return;
}
// 檢查目錄
File uploadDir = new File(savePath);
if (!uploadDir.isDirectory()) {
out.println(getError("上傳目錄不存在。"));
return;
}
// 檢查目錄寫許可權
if (!uploadDir.canWrite()) {
out.println(getError("上傳目錄沒有寫許可權。"));
return;
}
String dirName = request.getParameter("dir");
if (dirName == null) {
dirName = "image";
}
if (!extMap.containsKey(dirName)) {
out.println(getError("目錄名不正確。"));
return;
}
// 創建文件夾
savePath += dirName + "/";
saveUrl += dirName + "/";
File saveDirFile = new File(savePath);
if (!saveDirFile.exists()) {
saveDirFile.mkdirs();
}
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
String ymd = sdf.format(new Date());
savePath += ymd + "/";
saveUrl += ymd + "/";
File dirFile = new File(savePath);
if (!dirFile.exists()) {
dirFile.mkdirs();
}
FileItemFactory factory = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(factory);
upload.setHeaderEncoding("UTF-8");
List items = upload.parseRequest(request);
Iterator itr = items.iterator();
while (itr.hasNext()) {
FileItem item = (FileItem) itr.next();
String fileName = item.getName();
if (!item.isFormField()) {
// 檢查文件大小
if (item.getSize() > maxSize) {
out.println(getError("上傳文件大小超過限制。"));
return;
}
// 檢查擴展名
String fileExt = fileName.substring(fileName.lastIndexOf(".") + 1).toLowerCase();
if (!Arrays.<String> asList(extMap.get(dirName).split(",")).contains(fileExt)) {
out.println(getError("上傳文件擴展名是不允許的擴展名。
只允許" + extMap.get(dirName) + "格式。"));
return;
}
SimpleDateFormat df = new SimpleDateFormat("yyyyMMddHHmmss");
String newFileName = df.format(new Date()) + "_" + new Random().nextInt(1000) + "." + fileExt;
try {
File uploadedFile = new File(savePath, newFileName);
item.write(uploadedFile);
} catch (Exception e) {
out.println(getError("上傳文件失敗。"));
return;
}
JSONObject obj = new JSONObject();
obj.put("error", 0);
obj.put("url", saveUrl + newFileName);
out.println(obj.toString());
}
}
} catch (FileUploadException e1) {
e1.printStackTrace();
}
}
private String getError(String message) {
JSONObject obj = new JSONObject();
obj.put("error", 1);
obj.put("message", message);
return obj.toString();
}
}
項目中依賴的主要jar:commons-fileupload-1.2.jar,commons-io-1.4.jar
主要的兩個js文件:jquery.min.js,jquery-form.js
下載地址:http://download.csdn.net/detail/xiaole0313/8984223

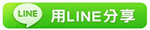
※python 進程實現多任務
※「並發編程」Future模式及JDK中的實現
TAG:程序員小新人學習 |