使用Python的matplotlib畫折線圖,柱狀圖,三維圖
因為在各種場景下需要各種實驗數據的對比圖像,有的中還要求dpi,這些在Python中的matplotlib中都可以實現,下面是總結的各種畫圖命令。
打包文件:https://download.csdn.net/download/pcb931126/10864654
"""
#Python中matplotlib中畫圖工具
#姓名:pcb
#時間:2018.12.20
"""
#引入畫圖所需要的庫文件
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from matplotlib.ticker import MultipleLocator
from mpl_toolkits.mplot3d import Axes3D
"""
畫折現圖
"""
# input_values=[1,2,3,4,5]
# squares=[1,4,9,16,25]
#
# plt.plot(input_values,squares,linewidth=5) #設置線寬
# plt.title("Square Number",fontsize=24) #設置圖題
# plt.xlabel("Value",fontsize=14) #設置x軸的標籤以及標籤的大小
# plt.ylabel("Square of value",fontsize=14) #設置y軸的標籤以及標籤的大小
# plt.tick_params(axis="both",labelsize=14) #設置刻度標記的大小
#
# plt.show()
"""
繪製散點圖
"""
# plt.scatter(2,4,s=200)
# plt.title("Square Number",fontsize=24) #設置圖題
# plt.xlabel("Value",fontsize=14) #設置x軸的標籤以及標籤的大小
# plt.ylabel("Square of value",fontsize=14) #設置y軸的標籤以及標籤的大小
# plt.tick_params(axis="both",which="major",labelsize=14) #設置刻度標記的大小
# plt.show()
"""
創建窗口子圖
設置子圖基本元素
"""
# x=np.arange(-5,5,0.1)
# y=x*3
# fig=plt.figure(num=1,figsize=(15,8),dpi=80) #開啟一個窗口,同時設置大小解析度,參數:窗口個數,窗口大小,解析度
# ax1=fig.add_subplot(2,1,1) #使用fig添加子圖,參數:行數、列數、第幾個
# ax2=fig.add_subplot(2,1,2) #使用fig添加子圖,參數:行數、列數、第幾個
#
# #####設置子圖窗口
# ax1.set_title("python_drawing",fontsize=12) #設置圖題以及字體大小
# ax1.set_xlabel("x_name",fontsize=12) #設置x軸的字體大小
# ax1.set_ylabel("y_name",fontsize=12) #設置y軸的字體大小
# plt.axis([-6,6,-10,10]) #設置橫縱坐標範圍,這個子圖中被分為一下兩個函數
# ax1.set_xlim(-5,5) #設置橫軸範圍,單獨給圖1設置x軸的範圍
# ax1.set_ylim(-10,10) #設置縱軸範圍,單獨給圖1設置y軸的範圍
# xmajorLocator = MultipleLocator(2) #定義橫向主刻度標籤的刻度差為2的倍數。就是隔幾個刻度才顯示一個標籤文本
# ymajorLocator = MultipleLocator(3) #定義縱向主刻度標籤的刻度差為3的倍數。就是隔幾個刻度才顯示一個標籤文本
# ax1.xaxis.set_major_locator(xmajorLocator) #應用定義的橫向主刻度格式
# ax1.yaxis.set_major_locator(ymajorLocator) #應用定義的縱向主刻度格式
# ax1.xaxis.grid(True,which="major") #x坐標軸網格使用主刻度格式
# ax1.yaxis.grid(True,which="major") #y坐標軸網格使用主刻度格式
# ax1.set_xticks([]) #去除坐標刻度
# ax1.set_xticks((-5,-3,-1,1,3,5)) #設置坐標刻度
#
# #設置刻度的顯示文本,rotation旋轉角度(>0順時針旋轉、<0逆時針旋轉),fontsize字體大小
# #ax1.set_xticklabels(labels=["x1","x2","x3","x4","x5"],rotation=-30,fontsize="small")
#
# #標誌marker為設置線的格式,具體標誌如下所示:
# """
# o』 圓圈
# 『.』 點
# 『D』 菱形
# 『s』 正方形
# 『h』 六邊形1
# 『*』 星號
# 『H』 六邊形2
# 『d』 小菱形
# 『_』 水平線
# 『v』 一角朝下的三角形
# 『8』 八邊形
# 『<』 一角朝左的三角形
# 『p』 五邊形
# 『>』 一角朝右的三角形
# 『,』 像素
# 『^』 一角朝上的三角形
# 『+』 加號
# 『 『 豎線
# 『None』,』』,』 『 無
# 『x』 X
#
# """
# #標誌color為顏色,具體標誌如下所示
# """
# b 藍色
# g 綠色
# r 紅色
# y 黃色
# c 青色
# k 黑色
# m 洋紅色
# w 白色
#
# """
#
# plot1=ax1.plot(x,y,marker="o",color="g",label="legend1")
#
#
# # #線圖:linestyle線性,alpha透明度,color顏色,label圖例文本
# plot2=ax1.plot(x,y,linestylex="--",alpha=0.5,color="r",label="legend2")
#
# ax1.legend(loc="upper left") #顯示圖例,plt.legend()
# ax1.text(2.8, 7, r"y=3*x") #指定位置顯示文字,plt.text()
# #
# # #添加標註,參數:注釋文本、指向點、文字位置、箭頭屬性
# ax1.annotate("important point", xy=(2, 6), xytext=(3, 1.5), arrowprops=dict(facecolor="black", shrink=0.05))
# #
# #顯示網格。which參數的值為major(只繪製大刻度)、minor(只繪製小刻度)、both,默認值為major。axis為"x","y","both"
# ax1.grid(b=True,which="major",axis="both",alpha= 0.5,color="skyblue",linestylex="--",linewidth=2)
#
# # # 在當前窗口添加一個子圖,rect=[左, 下, 寬, 高],是使用的絕對布局,不和以存在窗口擠占空間
# axes1 = plt.axes([.2, .3, .1, .1], facecolor="y")
# #
# # # 在子圖上畫圖
# axes1.plot(x,y)
#
# #savefig保存圖片,dpi解析度,bbox_inches子圖周邊白色空間的大小
# plt.savefig("aa.png",dpi=400,bbox_inches="tight")
#
# #打開窗口,對於方法1創建在窗口一定繪製,對於方法2方法3創建的窗口,若坐標系全部空白,則不繪製
# plt.show()
"""
極坐標圖
"""
# fig=plt.figure(2) #新開啟一個窗口
# ax1=fig.add_subplot(1,2,1,polar=True) #啟動一個窗口的極坐標子圖
# theta=np.arange(0,2*np.pi,0.02) #角度數列值
# ax1.plot(theta,2*np.ones_like(theta),lw=2) #畫圖,參數:角度,半徑,線寬
# ax1.plot(theta,theta/6,linestylex="--",lw=2) #畫圖,參數:角度,半徑,linestyle樣式,lw線寬
#
# ax2=fig.add_subplot(1,2,2,polar=True) #啟動一個極坐標子圖
# ax2.plot(theta,np.cos(5*theta),linestylex="--",lw=2)
# ax2.plot(theta,2*np.cos(4*theta),lw=2)
# ax2.set_rgrids(np.arange(0.2,0.2,0.2),angle=45) #距離網格軸,軸線刻度和顯示位置
# ax2.set_thetagrids([0,45,90]) #角度網格軸,範圍0-360度
# plt.savefig("11.png",dpi=400,bbox_inches="tight")
# plt.show()
"""
柱狀圖
"""
# plt.figure(3)
# x_index=np.arange(5) #柱的索引
# x_data=("A","B","C","D","E")
# y1_data=(20,35,30,35,27)
# y2_data=(25,32,34,20,25)
# bar0.35 #定義一個數字代表柱的寬度
# rects1 = plt.bar(x_index, y1_data, width=bar_width,alpha=0.4, color="b",label="legend1") #參數:左偏移、高度、柱寬、透明度、顏色、圖例
# rects2 = plt.bar(x_index + bar_width, y2_data, width=bar_width,alpha=0.5,color="r",label="legend2") #參數:左偏移、高度、柱寬、透明度、顏色、圖例
#
# #關於左偏移,不用關心每根柱的中心不中心,因為只要把刻度線設置在柱的中間就可以了
# plt.xticks(x_index + bar_width/2, x_data) #x軸刻度線
# plt.legend() #顯示圖例
# plt.tight_layout() #自動控制圖像外部邊緣,此方法不能夠很好的控制圖像間的間隔
# plt.savefig("11.png",dpi=400,bbox_inches="tight")
# plt.show()
"""
直方圖
"""
# fig,(ax0,ax1) = plt.subplots(nrows=2,figsize=(9,6)) #在窗口上添加2個子圖
# sigma = 1 #標準差
# mean = 0 #均值
# x=mean+sigma*np.random.randn(10000) #正態分布隨機數
# ax0.hist(x,bins=20,density=False,histtype="bar",facecolor="yellowgreen",alpha=0.75,rwidth=0.8) #normed是否歸一化,histtype直方圖類型,facecolor顏色,alpha透明度
# ax1.hist(x,bins=20,density=False,histtype="bar",facecolor="pink",alpha=0.75,cumulative=False,rwidth=0.8) #bins柱子的個數,cumulative是否計算累加分布,rwidth柱子寬度
# plt.savefig("12.png",dpi=400,bbox_inches="tight")
# plt.show() #所有窗口運行
"""
散點圖
"""
# fig = plt.figure(4) #添加一個窗口
# ax =fig.add_subplot(1,1,1) #在窗口上添加一個子圖
# x=np.random.random(100) #產生隨機數組
# y=np.random.random(100) #產生隨機數組
# ax.scatter(x,y,c="y",alpha=0.5,facecolors="none") #x橫坐標,y縱坐標,s圖像大小,c顏色,marker圖片,lw圖像邊框寬度
# plt.show() #所有窗口運行
"""
三維圖
"""
# fig = plt.figure(5)
# ax=fig.add_subplot(1,1,1,projection="3d") #繪製三維圖
#
# x,y=np.mgrid[-2:2:20j,-2:2:20j] #獲取x軸數據,y軸數據
# z=x*np.exp(-x**2-y**2) #獲取z軸數據
#
# ax.plot_surface(x,y,z,rstride=2,cstride=1,cmap=plt.cm.coolwarm,alpha=0.8) #繪製三維圖表面
# ax.set_xlabel("x-name") #x軸名稱
# ax.set_ylabel("y-name") #y軸名稱
# ax.set_zlabel("z-name") #z軸名稱
# plt.savefig("12.png",dpi=400,bbox_inches="tight")
# plt.show()
"""
畫矩形,多邊形、圓和橢圓
"""
# fig = plt.figure(6) #創建一個窗口
# ax=fig.add_subplot(1,1,1) #添加一個子圖
# rect1 = plt.Rectangle((0.1,0.2),0.2,0.3,color="r") #創建一個矩形,參數:(x,y),width,height
# circ1 = plt.Circle((0.7,0.2),0.15,color="r",alpha=0.3) #創建一個橢圓,參數:中心點,半徑,默認這個圓形會跟隨窗口大小進行長寬壓縮
# pgon1 = plt.Polygon([[0.45,0.45],[0.65,0.6],[0.2,0.6]]) #創建一個多邊形,參數:每個頂點坐標
#
# ax.add_patch(rect1) #將形狀添加到子圖上
# ax.add_patch(circ1) #將形狀添加到子圖上
# ax.add_patch(pgon1) #將形狀添加到子圖上
# plt.savefig("13.png",dpi=400,bbox_inches="tight")
# fig.canvas.draw() #子圖繪製
# plt.show()
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
畫圖結果如下:
柱狀圖
三維圖
折線圖
其他圖模塊圖
---------------------
作者:pcb931126
原文:https://blog.csdn.net/pcb931126/article/details/85124369

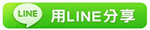
※Scrapy 入門教程
※如何修改request參數值並應用到springMvc中
TAG:程序員小新人學習 |