使用Node.js構建互動式命令行工具
使用 Node.js 構建一個根據詢問創建文件的命令行工具。
當用於構建命令行界面(CLI)時,Node.js 十分有用。在這篇文章中,我將會教你如何使用 Node.js 來構建一個問一些問題並基於回答創建一個文件的命令行工具。
開始
首先,創建一個新的 npm 包(NPM 是 JavaScript 包管理器)。
mkdir my-script
cd my-script
npm init
NPM 將會問一些問題。隨後,我們需要安裝一些包。
npm install --save chalk figlet inquirer shelljs
這是我們需要的包:
- Chalk:正確設定終端的字元樣式
- Figlet:使用普通字元製作大字母的程序(LCTT 譯註:使用標準字元,拼湊出圖片)
- Inquirer:通用互動式命令行用戶界面的集合
- ShellJS:Node.js 版本的可移植 Unix Shell 命令行工具
創建一個 index.js 文件
現在我們要使用下述內容創建一個 index.js 文件。
#!/usr/bin/env node
const inquirer = require("inquirer");
const chalk = require("chalk");
const figlet = require("figlet");
const shell = require("shelljs");
規劃命令行工具
在我們寫命令行工具所需的任何代碼之前,做計劃總是很棒的。這個命令行工具只做一件事:創建一個文件。
它將會問兩個問題:文件名是什麼以及文件後綴名是什麼?然後創建文件,並展示一個包含了所創建文件路徑的成功信息。
// index.js
const run = async () => {
// show script introduction
// ask questions
// create the file
// show success message
};
run();
第一個函數只是該腳本的介紹。讓我們使用 chalk 和 figlet 來把它完成。
const init = () => {
console.log(
chalk.green(
figlet.textSync("Node JS CLI", {
font: "Ghost",
horizontalLayout: "default",
verticalLayout: "default"
})
)
);
}
const run = async () => {
// show script introduction
init();
// ask questions
// create the file
// show success message
};
run();
然後,我們來寫一個函數來問問題。
const askQuestions = () => {
const questions = [
{
name: "FILENAME",
type: "input",
message: "What is the name of the file without extension?"
},
{
type: "list",
name: "EXTENSION",
message: "What is the file extension?",
choices: [".rb", ".js", ".php", ".css"],
filter: function(val) {
return val.split(".")[1];
}
}
];
return inquirer.prompt(questions);
};
// ...
const run = async () => {
// show script introduction
init();
// ask questions
const answers = await askQuestions();
const { FILENAME, EXTENSION } = answers;
// create the file
// show success message
};
注意,常量 FILENAME 和 EXTENSIONS 來自 inquirer 包。
下一步將會創建文件。
const createFile = (filename, extension) => {
const filePath = `${process.cwd()}/${filename}.${extension}`
shell.touch(filePath);
return filePath;
};
// ...
const run = async () => {
// show script introduction
init();
// ask questions
const answers = await askQuestions();
const { FILENAME, EXTENSION } = answers;
// create the file
const filePath = createFile(FILENAME, EXTENSION);
// show success message
};
最後,重要的是,我們將展示成功信息以及文件路徑。
const success = (filepath) => {
console.log(
chalk.white.bgGreen.bold(`Done! File created at ${filepath}`)
);
};
// ...
const run = async () => {
// show script introduction
init();
// ask questions
const answers = await askQuestions();
const { FILENAME, EXTENSION } = answers;
// create the file
const filePath = createFile(FILENAME, EXTENSION);
// show success message
success(filePath);
};
來讓我們通過運行 node index.js 來測試這個腳本,這是我們得到的:
完整代碼
下述代碼為完整代碼:
#!/usr/bin/env node
const inquirer = require("inquirer");
const chalk = require("chalk");
const figlet = require("figlet");
const shell = require("shelljs");
const init = () => {
console.log(
chalk.green(
figlet.textSync("Node JS CLI", {
font: "Ghost",
horizontalLayout: "default",
verticalLayout: "default"
})
)
);
};
const askQuestions = () => {
const questions = [
{
name: "FILENAME",
type: "input",
message: "What is the name of the file without extension?"
},
{
type: "list",
name: "EXTENSION",
message: "What is the file extension?",
choices: [".rb", ".js", ".php", ".css"],
filter: function(val) {
return val.split(".")[1];
}
}
];
return inquirer.prompt(questions);
};
const createFile = (filename, extension) => {
const filePath = `${process.cwd()}/${filename}.${extension}`
shell.touch(filePath);
return filePath;
};
const success = filepath => {
console.log(
chalk.white.bgGreen.bold(`Done! File created at ${filepath}`)
);
};
const run = async () => {
// show script introduction
init();
// ask questions
const answers = await askQuestions();
const { FILENAME, EXTENSION } = answers;
// create the file
const filePath = createFile(FILENAME, EXTENSION);
// show success message
success(filePath);
};
run();
使用這個腳本
想要在其它地方執行這個腳本,在你的 package.json 文件中添加一個 bin 部分,並執行 npm link:
{
"name": "creator",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo "Error: no test specified" && exit 1",
"start": "node index.js"
},
"author": "",
"license": "ISC",
"dependencies": {
"chalk": "^2.4.1",
"figlet": "^1.2.0",
"inquirer": "^6.0.0",
"shelljs": "^0.8.2"
},
"bin": {
"creator": "./index.js"
}
}
執行 npm link 使得這個腳本可以在任何地方調用。
這就是是當你運行這個命令時的結果。
/usr/bin/creator -> /usr/lib/node_modules/creator/index.js
/usr/lib/node_modules/creator -> /home/hugo/code/creator
這會連接 index.js 作為一個可執行文件。這是完全可能的,因為這個 CLI 腳本的第一行是 #!/usr/bin/env node。
現在我們可以通過執行如下命令來調用。
$ creator
總結
正如你所看到的,Node.js 使得構建一個好的命令行工具變得非常簡單。如果你希望了解更多內容,查看下列包。
- meow:一個簡單的命令行助手工具
- yargs:一個命令行參數解析工具
- pkg:將你的 Node.js 程序包裝在一個可執行文件中。
在評論中留下你關於構建命令行工具的經驗吧!
作者:Hugo Dias
原文:http://developer.51cto.com/art/201901/589669.htm

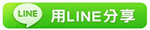
※Crunch團隊分享SpringCloud微服務的使用經驗
※Facebook是如何防止消息系統在新年前夕發生崩潰?
TAG:程序員小新人學習 |